Abs() method is a function of System.Math class which is used for finding the absolute value of any given number. It is a mathematical function and used to calculate the distance between 0 to given number. In this tutorial, we will learn Abs() method in detail using the simple programming example with dotnet6.0 and C#10
For better understanding, you can call Abs() method as Absolute method. This method can be overloaded by passing different types of parameters in it. If you want to find absolute value of any decimal number, just pass the decimal parameter in Abs method. You can use the following types of parameters to overload Math.Abs() function.
- Math.Abs(Decimal) - It is used for finding the absolute value of decimal number.
- Math.Abs(Double) - It is used for finding the absolute value of double-precision floating-point number.
- Math.Abs(Int16) - It is used for finding the absolute value of 16-bit integer value.
- Math.Abs(Int32) - It is used for finding the absolute value of 32-bit integer value.
- Math.Abs(Int64) - It is used for finding the absolute value of 64-bit integer value.
- Math.Abs(SByte) - It is used for finding the absolute value of 8-bit integer value.
- Math.Abs(Single) - It is used for finding the absolute value of single-precision floating-point number.
What is Absolute system in Math?
Most probably you have learned how to find Absolute value of any given number in your high school or middle school. Just to remind you about absolute system in math, I am giving a short overview of this.
Absolute value is just a distance between 0 to given number. Let’s clear it with example.
- The distance between 0 to 4 is 4 in the numerical line. So, absolute value of 4 is 4.
- The distance between 0 to -4 is also 4, so the absolute value of -4 is 4.
Absolute value is always a positive number.
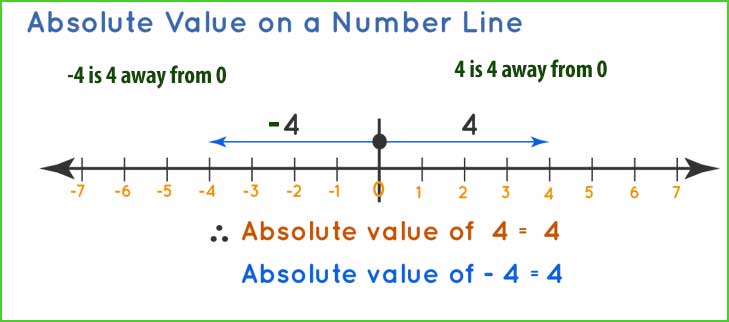
Math.Abs(Decimal)
Definition: As mentioned above this method is used to find absolute value of any given decimal number.
Declare: Public static decimal Abs (decimal value);
Parameter: decimal value
Return: Decimal value
Example 1: Simple Program
Console.WriteLine("Absolute Value of -5.12M is " + Math.Abs(-5.12M));
Output:
Absolute Value of -5.12M is 5.12
Example 2: Example with Array
decimal[] dcml = {15.23M, -11.523M, -87.42M, 0M, -5M, -0M, -123.55M}; foreach (decimal item in dcml) { Console.WriteLine($"Absolute value of {item} is = {Math.Abs(item)}"); }
Output
Absolute value of 15.23 is = 15.23 Absolute value of -11.523 is = 11.523 Absolute value of -87.42 is = 87.42 Absolute value of 0 is = 0 Absolute value of -5 is = 5 Absolute value of 0 is = 0 Absolute value of -123.55 is = 123.55
Example 3: Example with User Input
Console.WriteLine("Enter a decimal number: "); string? value = Console.ReadLine(); decimal dcml; if(decimal.TryParse(value, out dcml)) { Console.WriteLine($"Absolute value of {dcml} is = {Math.Abs(dcml)}"); } else { Console.WriteLine("The number is not decimal"); }
Output:
Enter a decimal number: -62.86 Absolute value of -62.86 is = 62.86
Math.Abs(Double)
Definition: When Math.Abs() method is overloaded with Double number, it finds absolute value of floating-point Double number. It takes parameter as Double number and returns value in Double numbers.
Declare: public static double Abs (double value);
Parameter: Double value
Return: Double value
Example 1: Simple Program
Console.WriteLine($"Absolute Value of {double.MinValue} is : " + Math.Abs(double.MinValue)); Console.WriteLine($"Absolute Value of {double.MaxValue} is : " + Math.Abs(double.MaxValue)); Console.WriteLine("Absolute Value of -5.12D is " + Math.Abs(-5.12D));
Output
Absolute Value of -1.7976931348623157E+308 is : 1.7976931348623157E+308 Absolute Value of 1.7976931348623157E+308 is : 1.7976931348623157E+308 Absolute Value of -5.12D is 5.12
Example 2: Example with Array
double[] dbl = { double.MinValue, -11.523D, -87.42D, 0D, -5D, -0D, -123.55D, double.MaxValue }; foreach (double item in dbl) { Console.WriteLine($"Absolute value of {item} is = {Math.Abs(item)}"); }
Output
Absolute value of -1.7976931348623157E+308 is = 1.7976931348623157E+308 Absolute value of -11.523 is = 11.523 Absolute value of -87.42 is = 87.42 Absolute value of 0 is = 0 Absolute value of -5 is = 5 Absolute value of -0 is = 0 Absolute value of -123.55 is = 123.55 Absolute value of 1.7976931348623157E+308 is = 1.7976931348623157E+308
Example 3: Example with User Input
Console.WriteLine("Enter a double number: "); string? value = Console.ReadLine(); double dbl; if (double.TryParse(value, out dbl)) { Console.WriteLine($"Absolute value of {dbl} is = {Math.Abs(dbl)}"); } else { Console.WriteLine("The number is not double"); }
Output
Enter a double number: -764235.534734568 Absolute value of -764235.534734568 is = 764235.534734568
Math.Abs(Int16), Math.Abs(Int32), Math.Abs(Int64)
Math.Abs(Int16)
It is used for finding absolute value of 16-bit signed integer. It uses short type data type.
Define:public static short Abs (short value);
Range:
int16.MinValue to int16.MaxValue
or,
short.MinValue to short.MaxValue
or,
-32768 to 32767
Exception: OverflowException
You will get OverflowException when you try to find absolute value of short.MinValue. This is because short.MinValue is greater than short.MaxValue. So, when you convert short minimum value into a positive number it raises OverflowException.
For exampleShort.MinValue is equal to -32768 and absolute value is 32768. But short type data type can hold value upto 32767 so, you will get OverflowException.
Example 1: Simple Program
Console.WriteLine($"Absolute Value of {Int16.MaxValue} is : " + Math.Abs(Int16.MaxValue)); Console.WriteLine("Absolute Value of -8675 is : " + Math.Abs(-8675)); // Console.WriteLine($"Absolute Value of {Int16.MinValue} is : " + Math.Abs(Int16.MinValue));
Output:
Absolute Value of 32767 is : 32767 Absolute Value of -8675 is : 8675
Example 2: Example with Array
short[] val = { short.MaxValue, -11, -87, 0, -5, -0, -123 }; foreach (short item in val) { Console.WriteLine($"Absolute value of {item} is = {Math.Abs(item)}"); }
Output
Absolute value of 32767 is = 32767 Absolute value of -11 is = 11 Absolute value of -87 is = 87 Absolute value of 0 is = 0 Absolute value of -5 is = 5 Absolute value of 0 is = 0 Absolute value of -123 is = 123
Example 3: Example with User Input
Console.WriteLine("Enter a short number between (-32768 to 32767: "); string? value = Console.ReadLine(); short val; if (short.TryParse(value, out val)) { Console.WriteLine($"Absolute value of {val} is = {Math.Abs(val)}"); } else { Console.WriteLine("The number is not a short number"); }
Output
Enter a short number between (-32768 to 32767: -5210 Absolute value of -5210 is = 5210
Math.Abs(Int32)
This method is used to find absolute value of 32-bit signed integer and it uses int type data type.
public static int Abs (int value);
Range:
Int32.MinValue to int32.MaxValue
or,
int.MinValue to int.MaxValue
or,
-2147483648 to 2147483647
Exception: OverflowException
Example 1: Simple Program
Console.WriteLine($"Absolute Value of {Int32.MaxValue} is : " + Math.Abs(Int32.MaxValue)); Console.WriteLine("Absolute Value of -5214225 is : " + Math.Abs(-5214225)); //Console.WriteLine($"Absolute Value of {Int32.MinValue} is : " + Math.Abs(Int32.MinValue));
Output
Absolute Value of 2147483647 is : 2147483647 Absolute Value of -5214225 is : 5214225
Example 2: Example with Array
int[] val = { int.MaxValue, -11, -87, 0, -5, -0, -123 }; foreach (int item in val) { Console.WriteLine($"Absolute value of {item} is = {Math.Abs(item)}"); }
Output
Absolute value of 2147483647 is = 2147483647 Absolute value of -11 is = 11 Absolute value of -87 is = 87 Absolute value of 0 is = 0 Absolute value of -5 is = 5 Absolute value of 0 is = 0 Absolute value of -123 is = 123
Example 3: Example with User Input
Console.WriteLine("Enter a number : "); string? value = Console.ReadLine(); int val; if (int.TryParse(value, out val)) { Console.WriteLine($"Absolute value of {val} is = {Math.Abs(val)}"); } else { Console.WriteLine("The number is not an integer number"); }
Output
Enter a number : -654897451 Absolute value of -654897451 is = 654897451
Math.Abs(Int64)
This method is used to find absolute value of 64-bit signed integer and it uses long type data type.
public static long Abs (long value);
Int64.MinValue to int64.MaxValue
or,
long.MinValue to long.MaxValue
or,
-9223372036854775808 to 9223372036854775807
Exception: OverflowException
Example 1: Simple Program
Console.WriteLine($"Absolute Value of {Int64.MaxValue} is : " + Math.Abs(Int64.MaxValue)); Console.WriteLine("Absolute Value of -5214225 is : " + Math.Abs(-5214225)); //Console.WriteLine($"Absolute Value of {Int64.MinValue} is : " + Math.Abs(Int64.MinValue));
Output
Absolute Value of 9223372036854775807 is : 9223372036854775807 Absolute Value of -5214225 is : 5214225
Example 2: Example with Array
Int64[] val = { Int64.MaxValue, -11, -87, 0, -5, -0, -123 }; foreach (long item in val) { Console.WriteLine($"Absolute value of {item} is = {Math.Abs(item)}"); }
Output
Absolute value of 9223372036854775807 is = 9223372036854775807 Absolute value of -11 is = 11 Absolute value of -87 is = 87 Absolute value of 0 is = 0 Absolute value of -5 is = 5 Absolute value of 0 is = 0 Absolute value of -123 is = 123
Example 3: Example with User Input
Console.WriteLine("Enter a number : "); string? value = Console.ReadLine(); long val; if (long.TryParse(value, out val)) { Console.WriteLine($"Absolute value of {val} is = {Math.Abs(val)}"); } else { Console.WriteLine("The number is not an Int64 number"); }
Output
Enter a number : -654854897545678654 Absolute value of -654854897545678654 is = 654854897545678654
Math.Abs(Sbyte)
Math.Abs(SByte) is used to find the absolute value of 8-bit signed integer.Syntax
public static sbyte Abs (sbyte value);
Range
-128 to 127
Exception: OverflowException
Example 1: Simple Program
Console.WriteLine($"Absolute Value of {SByte.MaxValue} is : " + Math.Abs(SByte.MaxValue)); Console.WriteLine("Absolute Value of -112 is : " + Math.Abs(-112)); //Console.WriteLine($"Absolute Value of {SByte.MinValue} is : " + Math.Abs(SByte.MinValue));
Output
Absolute Value of 127 is : 127 Absolute Value of -112 is : 112
Example 2: Example with Array
SByte[] val = { SByte.MaxValue, -11, -87, 0, -5, -0, -123 }; foreach (SByte item in val) { Console.WriteLine($"Absolute value of {item} is = {Math.Abs(item)}"); }
Output
Absolute value of 127 is = 127 Absolute value of -11 is = 11 Absolute value of -87 is = 87 Absolute value of 0 is = 0 Absolute value of -5 is = 5 Absolute value of 0 is = 0 Absolute value of -123 is = 123
Example 3: Example with User Input
Console.WriteLine("Enter a number : "); string? value = Console.ReadLine(); SByte val; if (SByte.TryParse(value, out val)) { Console.WriteLine($"Absolute value of {val} is = {Math.Abs(val)}"); } else { Console.WriteLine("The number is not an SByte number"); }
Output
Enter a number : -108 Absolute value of -108 is = 108
Math.Abs(Single)
The Math.Abs(Single) method is used for finding the absolute value of single precision floating point number.
Syntaxpublic static float Abs (float value);
Range
Example1:
Console.WriteLine($"Absolute Value of {Single.MaxValue} is : " + Math.Abs(Single.MaxValue)); Console.WriteLine("Absolute Value of -65456.2 is : " + Math.Abs(-65456.2)); Console.WriteLine($"Absolute Value of {Single.MinValue} is : " + Math.Abs(Single.MinValue));
Output
Absolute Value of 3.4028235E+38 is : 3.4028235E+38 Absolute Value of -65456.2 is : 65456.2 Absolute Value of -3.4028235E+38 is : 3.4028235E+38
Example 2: Example with Array
float[] val = { Single.MaxValue, -11.5F, -87.9F, 0.8F, -5.5F, -0.2F, -123.8F }; foreach (float item in val) { Console.WriteLine($"Absolute value of {item} is = {Math.Abs(item)}"); }
Output
Absolute value of 3.4028235E+38 is = 3.4028235E+38 Absolute value of -11.5 is = 11.5 Absolute value of -87.9 is = 87.9 Absolute value of 0.8 is = 0.8 Absolute value of -5.5 is = 5.5 Absolute value of -0.2 is = 0.2 Absolute value of -123.8 is = 123.8
Example 3: Example with User Input
Console.WriteLine("Enter a number : "); string? value = Console.ReadLine(); Single val; if (Single.TryParse(value, out val)) { Console.WriteLine($"Absolute value of {val} is = {Math.Abs(val)}"); } else { Console.WriteLine("The number is not a Single number"); }
Output
Enter a number : -652413.5 Absolute value of -652413.5 is = 652413.5
Summary
In this tutorial, we learned how to find absolute value of any given number in C# using the Math.Abs() method. In the next tutorial, we will learn about Math.Ceiling() method.