- What is
Delegates
? - What is
Lambda Expression
? - Delegates anonymous method
- Lambda Expression Programming Example
In order to understand LINQ well, you need to understand 2 topics very clearly.
1. Delegates2. Lambda Expression
In order to learn Lambda Expression you need to understand Delegates first. So, this is the chain of learning LINQ.
So, in this chapter, we will covers basics of all 3 areas with easy, comparative and beautiful programming example.
What is Delegate?
At first, if you are absolutely new in Delegate, then you can learn Delegates in easy way in this article.
C# Delegate Tutorial With Easy Example
Definition: A delegate is a class that represents the reference of methods with same signature (same parameter type and same return type). It is just like a pointer in C and C++ that contains memory location of attached variable. The difference between Delegates and Pointers is, Delegates is Type Safe, Thread Safe and secure than pointer.
Simple Programming Example of Delegate
Example 1: This is the simple delegate example that returns square of number.
using System; namespace LambdaExpression { class Program { public delegate int Square(int num); static void Main(string[] args) { Square sq = delegate (int num) { return num * num; }; Console.WriteLine(sq(5).ToString()); Console.ReadLine(); } } }Output: 25
Example 2: This is the same example but in a different way.
using System; namespace LambdaExpression { class Program { //Using Delegate public delegate int Square(int num); static int SquareNumber(int num) { return(Convert.ToInt32(num * num)); } static void Main(string[] args) { Square sq = SquareNumber; Console.WriteLine(sq(5).ToString()); Console.ReadLine(); } } }
Output: 25
Let’s understand this code
line 8: Created a named delegateSquare
that requires an integer type parameter.Line 10: Created a function with same signature as delegate that takes an integer number as input and returns square of the number.
Line 17: Attached
SquareNumber
function to delegate Square
and used it for generating square of the number.As you can see that I have created delegate Square
for attaching method in it. Now, I will discuss about 2 predefined delegate type that a programmer can use it and reuse it without creating and named delegate. These are Func<int, int>
and Action<int, int>
Let's see the same example using Func<int, int>
delegate type.
using System; namespace LambdaExpression { class Program { //Using Predefined Delegate Function static int SquareNumber(int num) { return(Convert.ToInt32(num * num)); } static void Main(string[] args) { Func<int, int> square = SquareNumber; Console.WriteLine(square(5).ToString()); Console.ReadLine(); } } }
Output: 25
As you can see that I have not created any named delegate. I used Func<int, int>
method directly to hold the reference of SquareNumber method.
Func<int, int>
is a built in generic delegate type that has zero or more input parameters and one out parameter. The last parameter is always an out parameter that returns the output value.
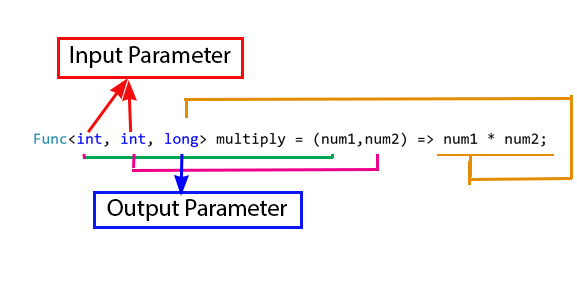
Lambda Expression
Lambda Expression
makes writing of delegate codes much easier, simpler and readable. Lambda Expression is the core building blocks of LINQ. Here, is the same example of SquareNumber with Lambda Expression.
using System; namespace LambdaExpression { class Program { //Using Lambda Expression static void Main(string[] args) { Func<int, int> square = (i => i * i); Console.WriteLine(square(5).ToString()); Console.ReadLine(); } } }
Output: 25
Let's understand
As you can see that the same code is written in few lines with the help of lambda expression. Lambda Expression is easy to learn and easy to use. This is the syntax of Lambda Expression.
In lambda expression, put input parameter in the left side of => operator and put statement block on the right side of => operator. For example:
x => x * x
x is a parameter that returns the square of x.
(x,y) => x * y
x and y is input parameter that returns multiplication of these number.
Learn Lambda Expression with Programming Example
Example 1: Here, I a am going to put simple example that multiply 2 integer input and returns result. I will write these program using 3 different techniques. 1. Simple function, 2. delegates and 3. lambda expression. So that you can easily compare and learn how lambda expression works.
Simple Function
//Using Normal Function public int Multiply(int num1, int num2) { return (Convert.ToInt32(num1 * num2)); } static void Main(string[] args) { Program p = new Program(); Console.WriteLine(p.Multiply(5,10).ToString()); Console.ReadLine(); }
Output: 50
Using Delegates
public delegate int Multiply(int num1, int num2); static void Main(string[] args) { Multiply mply = delegate (int num1, int num2) { return num1 * num2; }; Console.WriteLine(mply(5,10).ToString()); Console.ReadLine(); }
Output: 50
Using Lambda Expression
static void Main(string[] args) { Func<int, int, long> multiply = (num1,num2) => num1 * num2; Console.WriteLine(multiply(5,10).ToString()); Console.ReadLine(); }
Output: 50
In this example, I have used predefined delegates Func<int, int, long> in which int, int is two input parameter and long is a return type value.
Example 2: Find Even Number between 0 to 100
In this example, I will find even number between 0 – 100 using normal function, using delegates and using lambda expression.
Using Normal Functionpublic bool FindEvenNumber(int num) { return (num % 2 == 0); } static void Main(String[] args) { Program p = new Program(); for(int i = 0; i <= 20; i++) { if(p.FindEvenNumber(i)) { Console.WriteLine(i); } } Console.ReadLine(); }
Output
0 2 4 6 8 10 12 14 16 18 20
Using Delegates
//Using Delegate public delegate bool FindEvenNumber(int num); public static bool EvenNumber(int num) { return(num % 2 == 0); } static void Main(string[] args) { FindEvenNumber number = EvenNumber; for(int i = 0; i <= 20; i++) { if(number(i)) { Console.WriteLine(i); } } Console.ReadLine(); }
Output
0 2 4 6 8 10 12 14 16 18 20
Using Lambda Expression
//Using Lambda Expression static void Main(string[] args) { Func<int, bool> EvenNumber = (i => i % 2 == 0); for(int i = 0; i <= 20; i++) { if(EvenNumber(i)) { Console.WriteLine(i.ToString()); } } Console.ReadLine(); }
Output
0 2 4 6 8 10 12 14 16 18 20