- How to Create PDF from DIV content in ASP.NET MVC5?
- How to Create and Download PDF?
- Simple programming example
Most of the time as a developer; you need to generate PDF and make them available to download for user. In this article, I will explain how can you print and create a PDF file of div section and show a button to download PDF File.
This tutorial contains following part:
- Add Reference of
itextsharp.xmlworker
- View –
Index()
view will be used for displaying and printing PDF file. - Controller – I will use
HomeController.cs
for writing code.
Step 1: Create a New MVC Project and Add a Reference of itextsharp.xmlworker
Create a new MVC project of any name as you want and follow the steps to add reference for itextsharp.xmlworker
assembly.
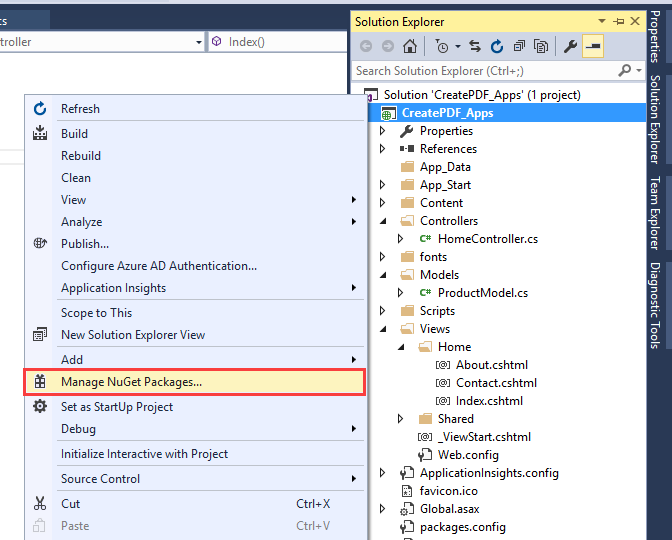
itextsharp.xmlworker
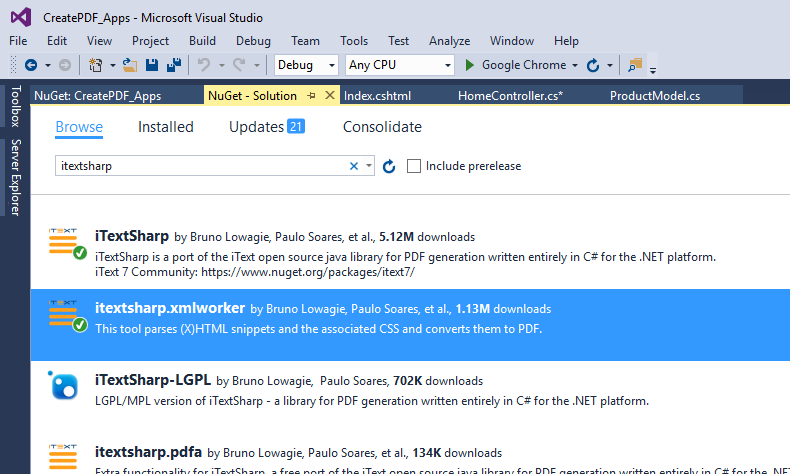
Step 2: View Page – Index.cshtml
Here, I am using Index.cshtml as view page. You can use any of your view page to print pdf.
@model IEnumerable<CreatePDF_Apps.Models.ProductModel>
@{
ViewBag.Title = "Home Page";
}
<div class="jumbotron">
<h1>CREATE PDF in ASP.Net MVC</h1>
</div>
<div class="row">
<div class="col-md-8">
<div id="PrintPDF">
<h1>This section is going to be printed in PDF</h1>
</div>
<br />
@using (Html.BeginForm("Export", "Home", FormMethod.Post))
{
<input type="hidden" name="ExportData" />
<input type="submit" id="btnSubmit" value="Export" />
}
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script>
<script type="text/javascript">
$(function () {
$("#btnSubmit").click(function () {
$("input[name='ExportData']").val($("#PrintPDF").html());
});
});
</script>
</div>
</div>
1. Use <div id="PrintPDF"> </div>
and all your content is going to be printed in PDF file.
2. Saves content in a hidden field control and used a submit button to download PDF File.
@using (Html.BeginForm("Export", "Home", FormMethod.Post)) { <input type="hidden" name="ExportData" /> <input type="submit" id="btnSubmit" value="Export" /> }
3. Used JQuery to fill hidden field control with div content.
@using (Html.BeginForm("Export", "Home", FormMethod.Post)) { <input type="hidden" name="ExportData" /> <input type="submit" id="btnSubmit" value="Export" /> }
Step 3: HomeController.cs
using System.Web.Mvc; using System.IO; using iTextSharp.text; using iTextSharp.text.pdf; using iTextSharp.tool.xml; namespace CreatePDF_Apps.Controllers { public class HomeController : Controller { public ActionResult Index() { return View(); } [HttpPost] [ValidateInput(false)] public FileResult Export(string ExportData) { using (MemoryStream stream = new System.IO.MemoryStream()) { StringReader reader = new StringReader(ExportData); Document PdfFile = new Document(PageSize.A4); PdfWriter writer = PdfWriter.GetInstance(PdfFile, stream); PdfFile.Open(); XMLWorkerHelper.GetInstance().ParseXHtml(writer, PdfFile, reader); PdfFile.Close(); return File(stream.ToArray(), "application/pdf", "ExportData.pdf"); } } } }
Output
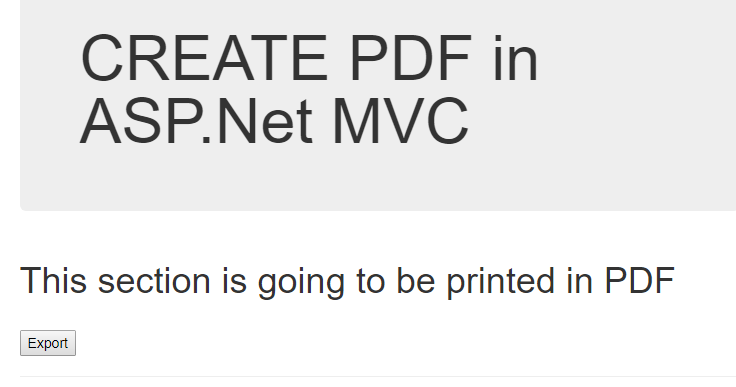
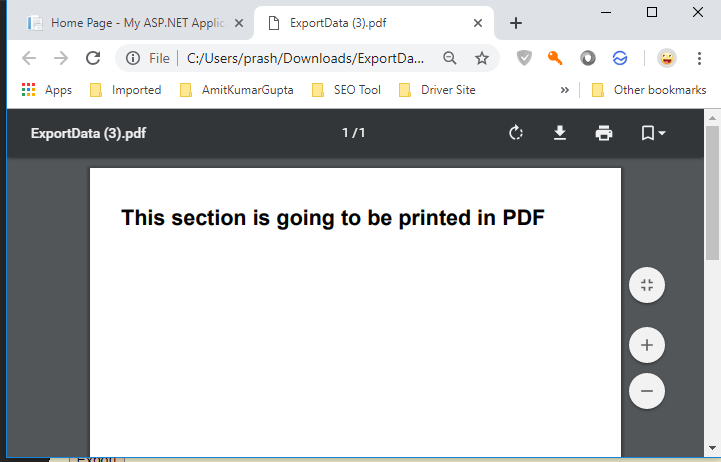
Summary
In this tutorial, I have simply explained how can you print a div in pdf in asp.net mvc5. In the next article, I will explain how to create a pdf from database table.