- How to upload image in MVC using C#?
- How to list image and data in Webgrid?
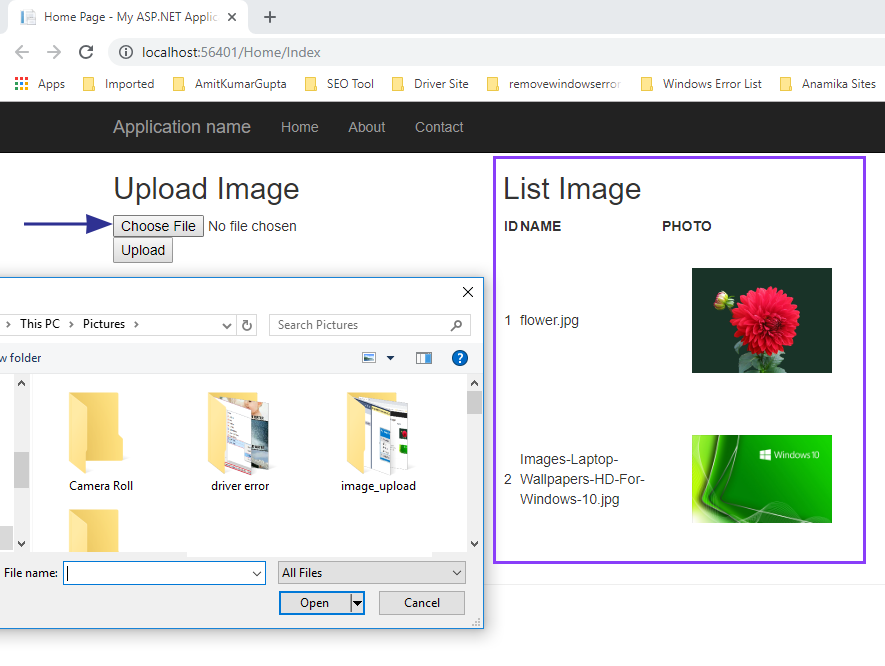
If you are looking for an article on how to upload and view image in WebGrid, then this article might be best choice for you. Here, I am going to upload and list image in WebGrid using very simple techniques.
Steps:
- Create a New Project
- Create Database, Table and Image Folder
- Add ADO.NET Entity Data Model
- Add a Controller
- Add a View
- Run the Project
Step 1: Create a New Project
Here, I am creating a project with the name Upload_Image.
- Open Visual Studio.
- Go to File > New > Project
- Select Visual C# in left pane and then select ASP.NET Web Application.
- Give Project Name Upload_Image and click OK.
- Select MVC in Template Window and set Authentication type: No Authentication. Click OK.
Step 2: Create Database, Table and upload Folder
In this part we will create a Database, a Table and a folder “upload” in Project folder. This Image folder will keep the uploaded image.
Create Database:
Upload_Image_DB
- Open Server Explorer by pressing CTRL + W or go to View > Server Explorer.
- Right click on Data Connections and select Create New SQL Server Database.
- Select your Server and then give Database Name as
Upload_Image_DB
. Click OK.
Create Table:
Image_Table
- Expand Upload_Image_DB in Solution Explorer and Right click on Table. Select Add New Table.
- Create a Table "Image_Table" as follows:
Script
CREATE TABLE [dbo].[Image_Table] ( [Id] INT NOT NULL PRIMARY KEY IDENTITY, [Title] NVARCHAR(200) NULL, [Image] NVARCHAR(MAX) NULL )
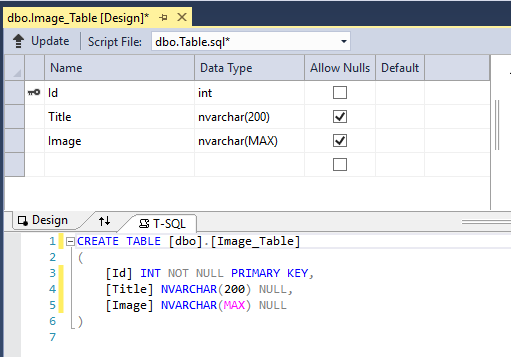
- You must set auto increment for Id field. Right click on Id column and select Properties.
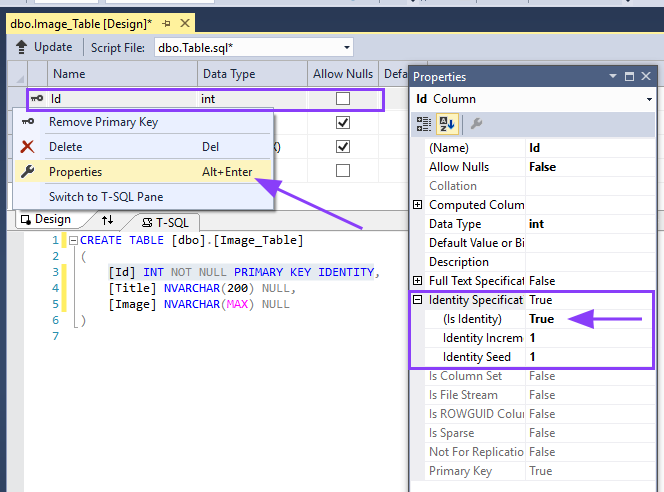
- In the Properties, Expand Identity Specification and then select (Is Identity) to True.
upload
- Go to Solution Explorer and Right click on Project Name > Add > New Folder.
- Set folder name as upload
Step 3: Add ADO.NET Entity Data Model
Now, we will add ADO.NET Entity Data Model. This model will create entity classes for database table and will give us a visual diagram of tables and relationship. This data model handles most of the configuration automatically and gives us easy way to work with database table.
- Go to Solution Explorer > Right click on Project > Add New Item.
- Select Data in Left Pane and then Select ADO.NET Entity Data Model. Give model name as:
ImageTableModel
. Click on Add. - Select EF Designer from Database in next wizard and click Next.
- In the next Window, click on New Connection button and then select your server and database. Give connection name as
Upload_Image_Constring
and then click Next. - In the next window, Select Table and then click on Finish button.
- You will see that Table diagram is created.
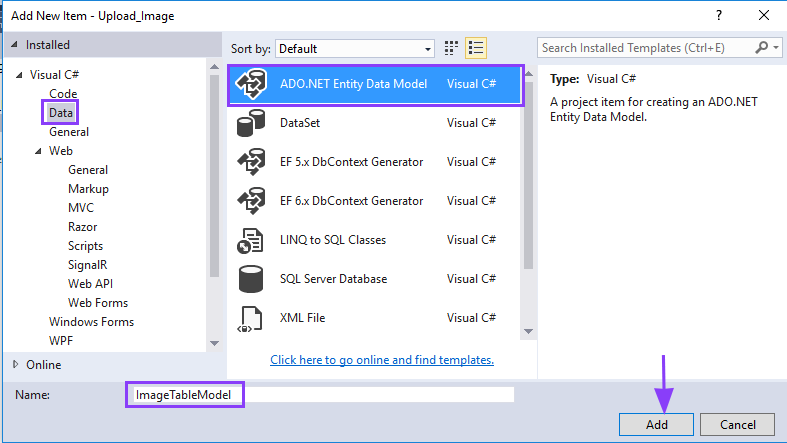
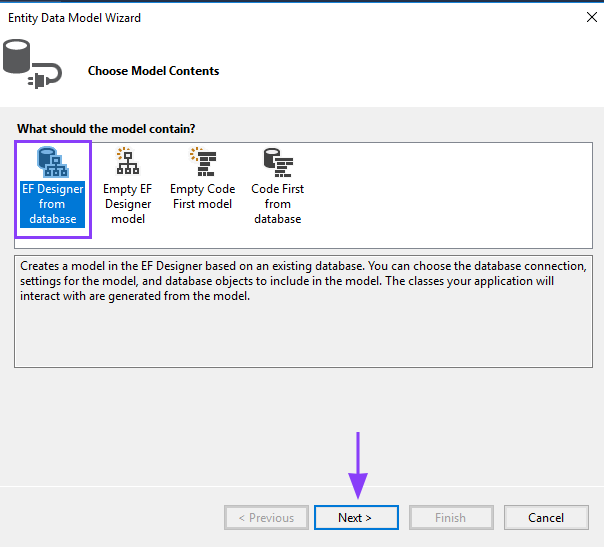
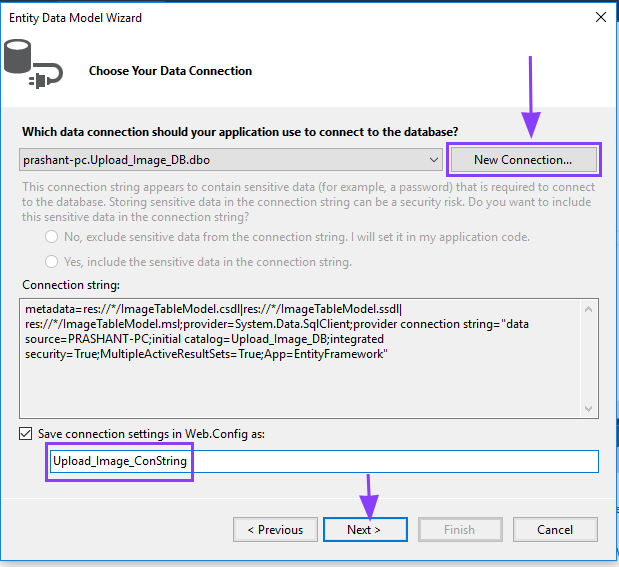
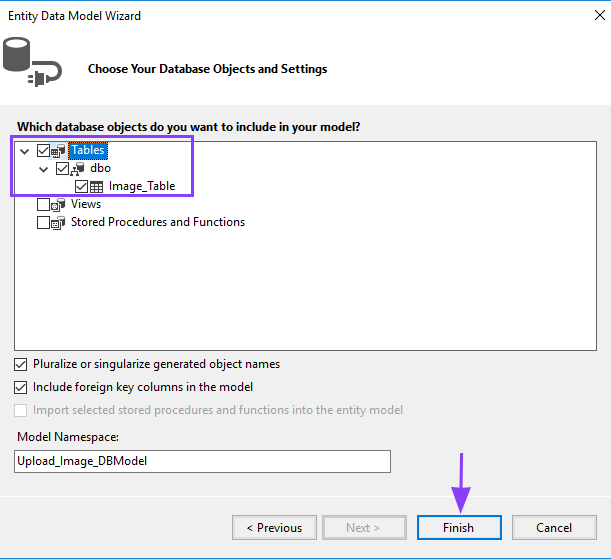
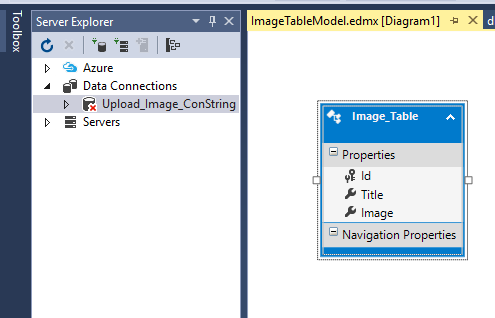
Step 4: Add a Controller
Till now, you have successfully created Database, Table and Entity Model. Now, this time to create an Action Method in HomeController and use entity data model to save, upload and retrieve image.
- Open
HomeController.cs
- Add following code in it.
using System.Linq; using System.Web; using System.Web.Mvc; using System.IO; namespace Upload_Image.Controllers { public class HomeController : Controller { // This method will list the table' row [HttpGet] public ActionResult Index() { Upload_Image_ConString connection = new Upload_Image_ConString(); return View(connection.Image_Table.ToList()); } // This method will upload the image and data to the folder and database [HttpPost] public ActionResult Index(HttpPostedFileBase file) { //Getting File Details string name = Path.GetFileName(file.FileName); string path = "~/upload/" + name; //Saving file to Folder file.SaveAs(Server.MapPath(path)); //Saving data to database Upload_Image_ConString connection = new Upload_Image_ConString(); connection.Image_Table.Add(new Image_Table { Title = name, Image = path }); connection.SaveChanges(); return RedirectToAction("Index"); } public ActionResult About() { ViewBag.Message = "Your application description page."; return View(); } public ActionResult Contact() { ViewBag.Message = "Your contact page."; return View(); } } }
Step 5: Adding View
After editing HomeController, you need to add FileUploader control in razor.
- Open Views > Home >
Index.cshtml
from solution explorer. - Paste the following code.
@model IEnumerable<Upload_Image.Image_Table> @{ ViewBag.Title = "Home Page"; WebGrid webGrid = new WebGrid(source: Model, canSort: false, canPage: false); } <div class="row"> <div class="col-md-4"> <h2>Upload Image</h2> <p> @using (Html.BeginForm("Index", "Home", FormMethod.Post, new { enctype = "multipart/form-data" })) { <input type="file" name="postedFile" /> <input type="submit" id="btnUpload" value="Upload" /> } <hr /> </p> </div> <div class="col-md-4"> <h2>List Image</h2> <p> @webGrid.GetHtml( htmlAttributes: new { @id = "WebGrid" }, columns: webGrid.Columns( webGrid.Column("Id", "ID"), webGrid.Column("Title", "NAME"), webGrid.Column("Image", "PHOTO", format:@<text><img alt="@item.Title" src="@Url.Content(item.Image)" style="width:200px; height:auto; padding:30px;" /></text>))) </p> </div> </div> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.8.3/jquery.min.js"></script> <link rel="stylesheet" href="https://ajax.googleapis.com/ajax/libs/jqueryui/1.8.24/themes/start/jquery-ui.css" /> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jqueryui/1.8.24/jquery-ui.min.js"></script>
Step 6: Run the Project
Press F5 to run the Project.
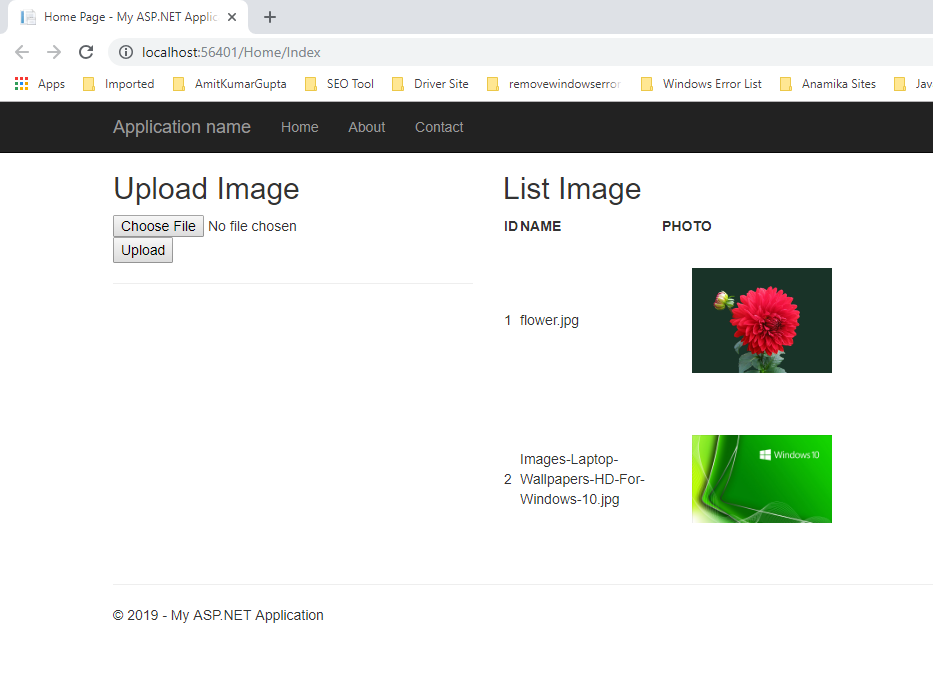
Summary
In this article, I explained how can you upload image in MVC C# and list them in WebGrid.