In this article you will learn
- How to Multiple File Upload in ASP.NET MVC with C#?
- Simple Programming Example on Multiple File Uploading
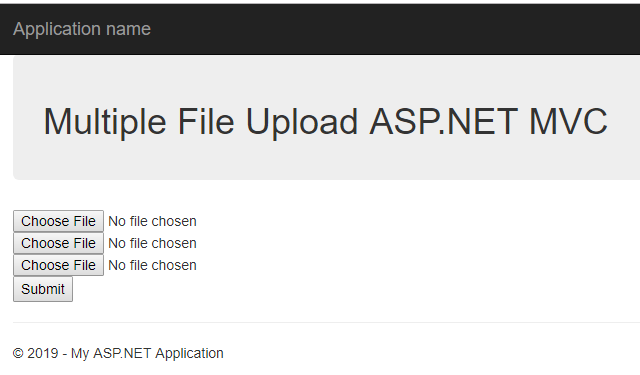
In the previous article, I have explained how to upload a single file in easy way and in this article, I am going to describe how can you insert multiple file upload button in a view page and upload all the file with just a single click.
Step 1: Create a New MVC Project. Open Visual Studio and Go to File > New > Project and select ASP.NET Web Application (.Net Framework).
Step 2: Open
Index.cshtml
View Page. Go to Solution Explorer > Views > Home > Index.cshtml
and write the following code.@{ ViewBag.Title = "Home Page"; } <div class="jumbotron"> <h1>Multiple File Upload ASP.NET MVC</h1> </div> <div class="row"> <div class="col-md-4"> <form action="" method="post" enctype="multipart/form-data"> <input type="file" name="files" id="file1" /> <input type="file" name="files" id="file2" /> <input type="file" name="files" id="file3" /> <input type="submit" /> </form> </div> </div>
Step 3: Open
HomeController.cs
and write following code.using System.Collections.Generic; using System.IO; using System.Web; using System.Web.Mvc; namespace SimpleFileUpload.Controllers { public class HomeController : Controller { [HttpGet] public ActionResult Index() { return View(); } //Multiple File Upload [HttpPost] public ActionResult Index(IEnumerable<HttpPostedFileBase> files) { foreach (var file in files) { if (file.ContentLength > 0) { var fileName = Path.GetFileName(file.FileName); var path = Path.Combine(Server.MapPath("~/App_Data/uploads"), fileName); file.SaveAs(path); } } return RedirectToAction("Index"); } } }
Step 4: Create a folder uploads inside App_Data. Right click on App_Data > Add > New Folder.
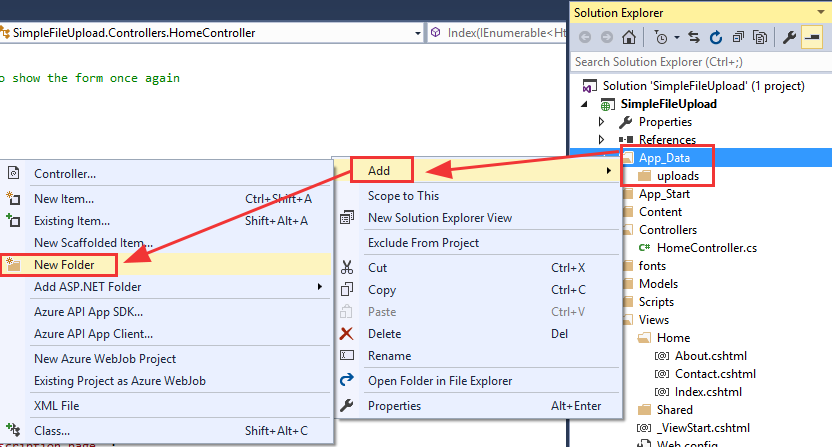
Step 5: Build and Run your Project.
Press CTRL + SHIFT + B to build the project and then Press CTRL + F5 to run the project.
Output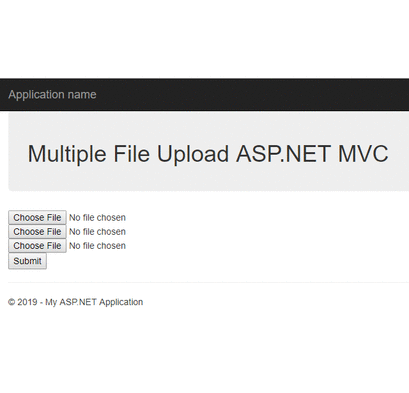
Summary
In this article, I put the example of uploading multiple files at once in ASP.NET MVC. This is very simple and basic example and if you are learning ASP.NET MVC then this article might help you a lot.