- What is single dimensional array?
- How to declare one dimensional array?
- How to use single dimensional array in C# programming?
The one dimensional array or single dimensional array in C# is the simplest type of array that contains only one row for storing data. It has single set of square bracket (“[]”). To declare single dimensional array in C#, you can write the following code.
string[] Books = new string[5];
The array age is a one dimensional array that contains only 5 elements in a single row.
Programming Example of One Dimensional Array:
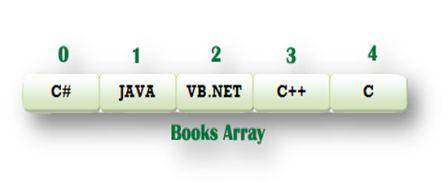
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace One_Dimensional_Array { class Program { static void Main(string[] args) { //Declaring single dimensional array string[] Books = new string[5]; Books[0] = "C#"; Books[1] = "Java"; Books[2] = "VB.NET"; Books[3] = "C++"; Books[4] = "C"; Console.WriteLine("All the element of Books array is:\n\n"); int i = 0; //Formatting Output Console.Write("\t1\t2\t3\t4\t5\n\n\t"); for (i = 0; i < 5; i++) { Console.Write("{0}\t", Books[i]); } Console.ReadLine(); } } }
Output
All the element of Books array is:
1 2 3 4 5
C# Java VB.NET C++ C
__
Summary
In this chapter you learned about single dimensional array in C#. You also learned how to store value and access value from single dimensional array. In next chapter you will learn about multi dimensional array in C#.