- How events return a value?
- Built in delegates in c#
- Programming Example
Built in Delegates in C#
In the previous chapter we learned to create custom events in c#. In this chapter you will know how to use in built delegates in c#. Microsoft provides 2 built in delegates to work with.
public delegate void EventHandler(object sender, EventArgs e); public delegate void EventHandler<TEventArgs>(object sender, TEventArgs e);
These built in delegates helps you to write event handling code with ease. With these delegates you can pass one or more than one value to event handler. When you raise event you must follow the discipline and pass required parameters to delegates.
Example 1:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace event_programming { //This is Subscriber Class class Program { static void Main(string[] args) { AddTwoNumbers a = new AddTwoNumbers(); //Event gets binded with delegates a.ev_OddNumber += EventMessage; a.Add(); Console.Read(); } //Delegates calls this method when event raised. static void EventMessage(object sender, EventArgs e) { Console.WriteLine("***Event Executed : This is Odd Number***"); } } //This is Publisher Class class AddTwoNumbers { public event EventHandler<EventArgs> ev_OddNumber; public void Add() { int result; result = 5 + 4; Console.WriteLine(result.ToString()); //Check if result is odd number then raise event if((result % 2 != 0) && (ev_OddNumber != null)) { ev_OddNumber(this, EventArgs.Empty); //Raised Event } } } }
9
***Event Executed : This is Odd Number***
_
Explanation
In this program, I have used built in delegates in c#.- Declare built in delegates
public event EventHandler<EventArgs> ev_OddNumber;
- Raised Event
ev_OddNumber(this, EventArgs.Empty);
this
denotes the current instance of class
EventArgs.Empty
tells that there is empty value in parameter. - Initialize Events in Main function
a.ev_OddNumber += EventMessage;
- Calls the methods with required parameters.
static void EventMessage(object sender, EventArgs e) { Console.WriteLine("***Event Executed : This is Odd Number***"); }
In the above program the events doesn't return any value. Now, look for the next modified program that returns a value with EventArgs.
Example 2
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace event_programming { class Program { static void Main(string[] args) { AddTwoNumbers a = new AddTwoNumbers(); a.ev_OddNumber += EventMessage; a.Add(); Console.Read(); } static void EventMessage(object sender, OddNumberEventArgs e) { Console.WriteLine("Event Executed : {0} is an Odd Number", e.sum); } } public class AddTwoNumbers { public event EventHandler<OddNumberEventArgs> ev_OddNumber; public void Add() { int result; result = 5 + 4; Console.WriteLine(result.ToString()); if((result % 2 != 0) && (ev_OddNumber != null)) { ev_OddNumber(this, new OddNumberEventArgs(result)); //Raised Event } } } //Creating Custom EventArgs public class OddNumberEventArgs : EventArgs { public OddNumberEventArgs(int result) { sum = result; } public int sum { get; set; } } }
Output
9
Event Executed : 9 is an Odd Number
_
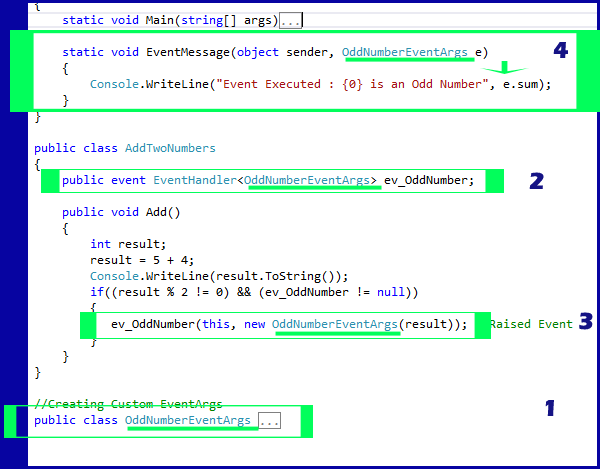
Let's understand this example.
- I have created a custom OddNumberEventArgs class that inherits the EventArgs. This class simply set the parameter value to sum variable.
public class OddNumberEventArgs : EventArgs { public OddNumberEventArgs(int result) { sum = result; } public int sum { get; set; } }
- Now, created event
ev_OddNumber
using this class.public event EventHandler<OddNumberEventArgs> ev_OddNumber;
- Pass required parameter when called this event.
ev_OddNumber(this, new OddNumberEventArgs(result)); //Raised Event
- Now in the
EventMessage()
function;OddNumberEventArgs e
has a value.static void EventMessage(object sender, OddNumberEventArgs e) { Console.WriteLine("Event Executed : {0} is an Odd Number", e.sum); }
Summary
In this chapter you learned about built in delegates and also learned how to return a value using custom EventArgs class. In the next chapter there are some easy exercise questions on Delegates and Events that will help you to understand event handling more clearly.