- What is Events?
- How Events work with Delegates?
- What is Publisher-Subscriber Model?
- How to use Events with Delegates?
What is Events?
Events are nothing just a user action. For example- When you click with the mouse – It is mouse click events.
- When you press any key in keyboard – It is KeyPress events
- When you refresh your webpage – It is page load events
- When you move mouse cursor – It is mouse hover events etc.
So when you take any action like a key press, mouse movements, clicks etc an event raised. Let me clear more about it. For example, you filled an online form and click on submit button.
- In the background
button_click()
event raised. - This event calls and execute an associated function
Submit_Click()
. - This function processes your request and submits page information to database.
How Events work with Delegates?
Delegates are used to reference a method. An Event is associated with Event Handler using Delegates. When an Event raise, it sends a signal to delegates and delegates executes the right function.
What is Publisher-Subscriber Model?
There are two parts in any event handling program. One part is Publisher that contains definition of events and delegates and another part is Subscriber that accepts the event and provides an event handler.
Important Fact about Events
- An Event is created using event keyword.
- An Event has no return type and it is always void.
- All events are based on delegates.
- All the published events must have a listening object.
- All Events should be defined starting with “On” keyword.
Before seeing the programming examples you must know the sequential steps to manipulate events.
Step 1: Define a Delegate
Step 2: Define an Event with same name of Delegates.
Step 3: Define an Event Handler that respond when event raised.
Step 4: You must have method ready for delegates.
Programming Example:
This program simply adds two numbers. Only condition is if the sum of number is odd it fires an event that print a message using delegates.
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace event_programming { //This is Subscriber Class class Program { static void Main(string[] args) { AddTwoNumbers a = new AddTwoNumbers(); //Event gets binded with delegates a.ev_OddNumber += new AddTwoNumbers.dg_OddNumber(EventMessage); a.Add(); Console.Read(); } //Delegates calls this method when event raised. static void EventMessage() { Console.WriteLine("********Event Executed : This is Odd Number**********"); } } //This is Publisher Class class AddTwoNumbers { public delegate void dg_OddNumber(); //Declared Delegate public event dg_OddNumber ev_OddNumber; //Declared Events public void Add() { int result; result = 5 + 4; Console.WriteLine(result.ToString()); //Check if result is odd number then raise event if((result % 2 != 0) && (ev_OddNumber != null)) { ev_OddNumber(); //Raised Event } } } }
Output:
9
********Event Executed : This is Odd Number**********
_
Explanation
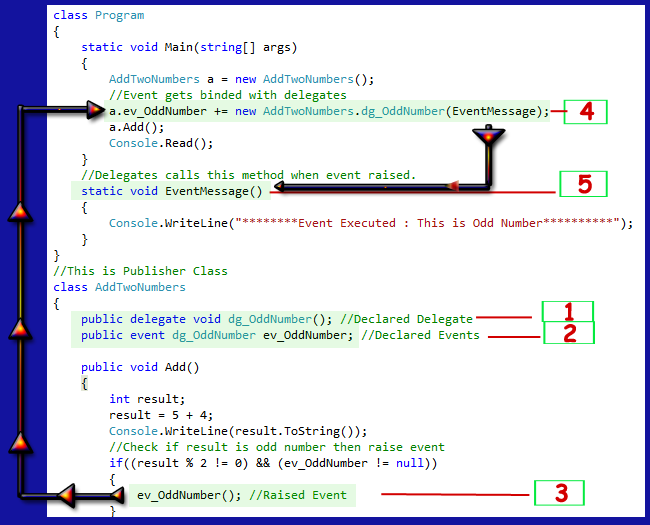
See this picture. There is some marking on the code. We will go through the marking and understand the process.
- Delegate Created
public delegate void dg_OddNumber();
- Event Created
public event dg_OddNumber ev_OddNumber;
ev_OddNumber()
event gets executed if the odd number is found.- In the AddTwoNumbers class there is a function
void Add()
that adds two numbers. If the sum of the number is Odd it raised anev_OddNumber
event.In the main function thisev_OddNumber
event handler calls the delegatesa.ev_OddNumber += new AddTwoNumbers.dg_OddNumber(EventMessage);
- Then finally delegate executes the function.
static void EventMessage() { Console.WriteLine("********Event Executed : This is Odd Number**********"); }
Summary
In this chapter you learned what is event programming in C# and how to use it in programming. It is the very basic programming example. You would have noticed that the event didn't return any value to event handler. How it would be nice if event sends the odd number to delegates so delegates could print this number along with message.
In the next chapter I have added some programming examples that will help you to understand built in delegates and event programming more clearly.