In this chapter you will learn:
- What is Queue<T> in C#?
- How to initialize it?
- Understand C# Queue with Programming Example
What is Queue<T>?
Queue Represents First In – First Out collection of objects. Let’s understand it with an easy example. Take an example of a line of the ticket counter. The person which comes first take ticket first. All the person wait according to their insertion order in line and when they reach the top of the line they get a ticket. In C# programming, Queue<T> does the same thing. The item which is inserted first can be accessed first.
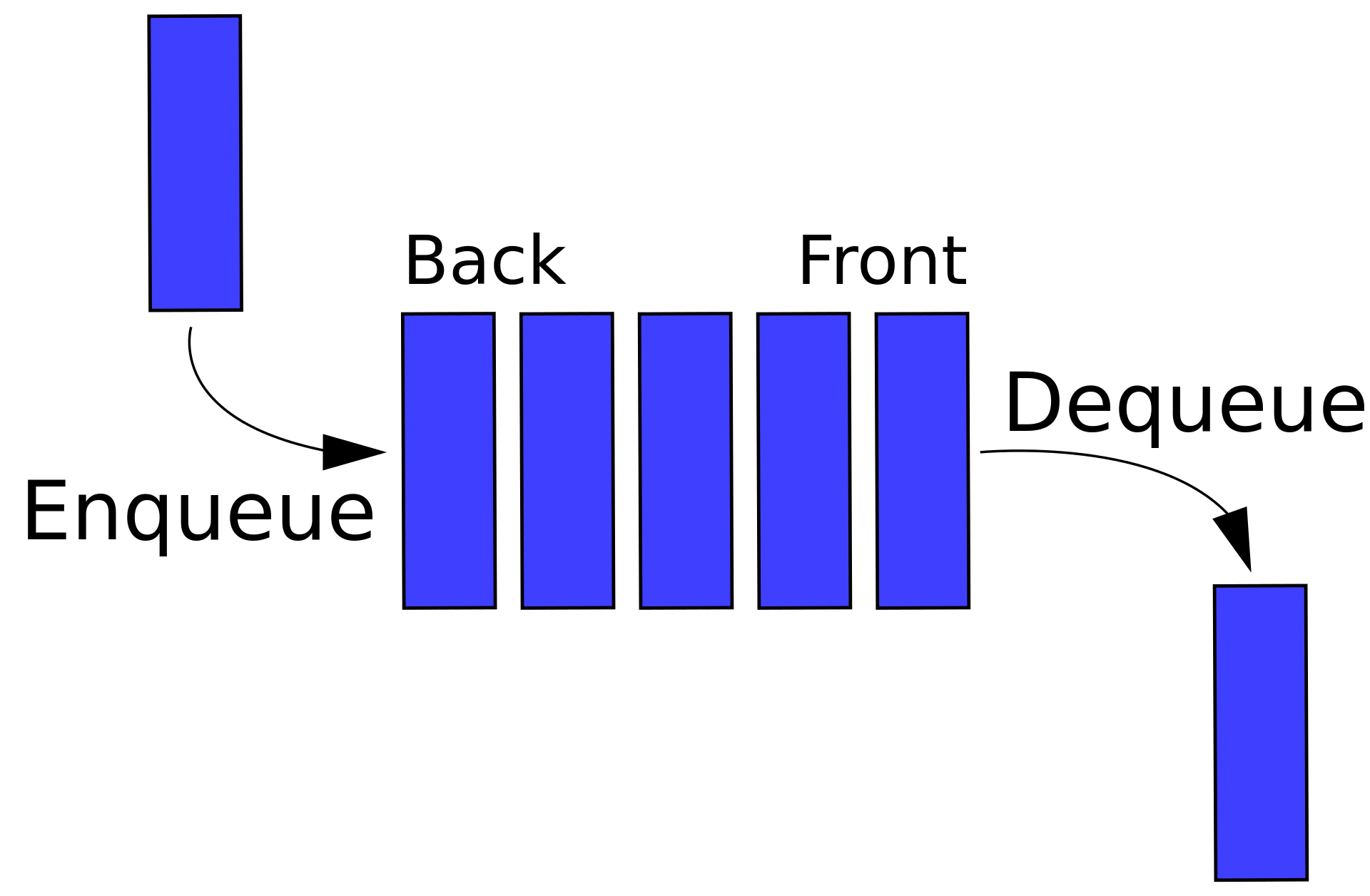
How to Initialize a Queue?
You can initialize queue as follows:Queue<string> numbers = new Queue<string>();
Properties
Properties | Description |
---|---|
Count |
Gets the number of elements contained in the Queue<T>. |
Methods
Methods | Description |
---|---|
Clear() |
Removes all objects from the Queue<T>. |
Contains(T) |
Determines whether an element is in the Queue<T>. |
CopyTo(T[], Int32) |
Copies the Queue<T> elements to an existing one-dimensional Array, starting at the specified array index. |
Dequeue() |
Removes and returns the object at the beginning of the Queue<T>. |
Enqueue(T) |
Adds an object to the end of the Queue<T>. |
Equals(Object) |
Determines whether the specified object is equal to the current object.(Inherited from Object.) |
Finalize() |
Allows an object to try to free resources and perform other cleanup operations before it is reclaimed by garbage collection.(Inherited from Object.) |
GetEnumerator() |
Returns an enumerator that iterates through the Queue<T>. |
GetHashCode() |
Serves as the default hash function. (Inherited from Object.) |
GetType() |
Gets the Type of the current instance.(Inherited from Object.) |
MemberwiseClone() |
Creates a shallow copy of the current Object.(Inherited from Object.) |
Peek() |
Returns the object at the beginning of the Queue<T> without removing it. |
ToArray() |
Copies the Queue<T> elements to a new array. |
ToString() |
Returns a string that represents the current object.(Inherited from Object.) |
TrimExcess() |
Sets the capacity to the actual number of elements in the Queue<T>, if that number is less than 90 percent of current capacity. |
Programming Example
using System; using System.Collections.Generic; namespace QueueExample { public class Program { public static void Main(string[] args) { Queue<string> student=new Queue<string>(); //Adding item in queue student.Enqueue("Mark"); student.Enqueue("Jack"); student.Enqueue("Sarah"); student.Enqueue("Smith"); student.Enqueue("Robbie"); print(student); //Removing Item Console.WriteLine("\nRemoving {0} from List. \nNew list is : ",student.Dequeue()); print(student); //Copy Array Item to Queue string[] city={"Newyork", "California","Las Vegas"}; Queue<string> citylist=new Queue<string>(city); Console.WriteLine("\nPrinting City List"); print(citylist); //Count items in Queue Console.WriteLine("Count{0}",citylist.Count); } public static void print(Queue<string> q) { foreach(string s in q) { Console.Write(s.ToString()+" | "); } } } }
Output
Mark | Jack | Sarah | Smith | Robbie |
Removing Mark from List.
New list is :
Jack | Sarah | Smith | Robbie |
Printing City List
Newyork | California | Las Vegas | Count3
_
Summary
In this chapter you learned Queue<T> in C# with complete programming example. In the next chapter you will learn Stack<T> in C# Generics.