- What is Private Access Specifier?
- What is the boundary of private access specifier in C#?
- How to use private access specifier in C# programming?
The private access specifiers restrict the member variable or function to be called outside of the parent class. A private function or variable cannot be called outside of the same class. It hides its member variable and method from other class and methods. However, you can store or retrieve the value from private access modifiers using get the set property. You will learn more about get set property in lateral chapter.
Example:
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Private_Access_Specifiers { class access { // String Variable declared as private private string name; public void print() // public method { Console.WriteLine("\nMy name is " + name); } } class Program { static void Main(string[] args) { access ac = new access(); Console.Write("Enter your name:\t"); // raise error because of its protection level ac.name = Console.ReadLine(); ac.print(); Console.ReadLine(); } } }
Output
Error 1: Private_Access_Specifiers.access.name' is inaccessible due to its protection level __
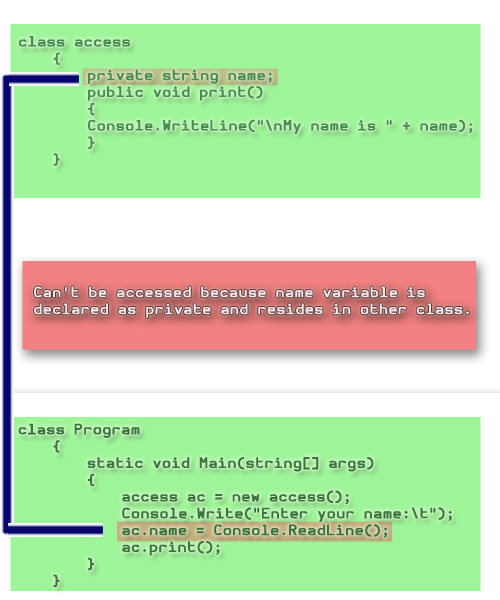
In the above example, you cannot call name variable outside the class because it is declared as private.
Summary
In this chapter you learned what is the private access specifier in C#? You also learned its scope and boundary and implementation in programming. In next chapter you will learn Protected Access Specifier in C#.