- What is Value type?
- What is difference between value type and reference type?
- How to pass value type parameter to a function?
As mentioned in the previous chapter, there are two types of the parameter that can be passed in function. One is Value type parameter and another is reference type parameter. Before understanding both types of parameters in C#, you will have to understand Value type and Reference type.
There are two ways to allocating space in memory. One is a value type and another is a Reference type. When you create int, char or float type variable, it creates value type memory allocation whereas when you create an object of a class, it creates reference type memory allocation.
Value Type: A value type variable directly contains data in the memory.Reference Type: A Reference type variable contains memory address of value.
Consider the following graph to make better sense of value type and reference type.
int Result; result=200;
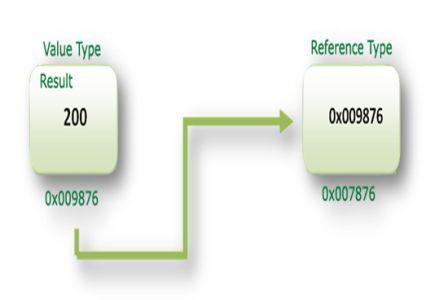
In the preceding example, the value type variable contains the value whereas a reference type variable contains the address of Result variable.
Value Type Parameter:
In value type parameter, the actual value gets passed to the function. Passing a value type variable as parameter means, you are passing the copy of the value.Programming Example
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace Value_Type { class Program { public static int qube(int num) { return num * num * num; } static void Main(string[] args) { int val, number; number = 5; //Passing the copy value of number variable val = Program.qube(number); Console.Write(val); Console.ReadLine(); } } }
Output
125
__
Summary
In this chapter you learned in details about value type parameter in C#. You also learned how to use value type parameter in c sharp programming. In next chapter you will learn about Reference Type Parameter in C#.