In this chapter, you will learn:
1. How to Add a View Pages in ASP.NET MVC 5?
2. How to Add a View Pages from Controller’s Action Method?
There are 2 most popular methods to adding a View Pages in ASP.NET MVC 5. 1. How to Add a View Pages in ASP.NET MVC 5?
2. How to Add a View Pages from Controller’s Action Method?
- Either you can directly add a View Page
- Or, Add a View Page from Controller.
1. View Pages must refer to a controller action method. If you have added a view page but didn’t create an action method in the controller, then you will get HTTP 404 Error. This is because, in MVC, Controller manages user request and returns a response to the user. So, if the action method isn’t available in a controller, the controller will return HTTP 404 Error message.
Let's understand it with a real example.
a. Suppose, you open the following link:localhost:1233/Home/Enquiry
Here,
localhost:1233
is your application or domain nameHome
is controller, andEnquiry
is Action Method.b. The request goes to
Home Controller
first and finds Enquiry
Action Method.c. If
Enquiry
Action method found in Home
Controller then it will look for Enquiry.cshtml
View Pages in Home
Folder.
d. You must remember that every Controller should have a folder in Views with the same name as controller name. And, every folder in View should have
.cshtml
view pages as the same name as an action method.
e. If there is
Enquiry
Action Method in Home
Controller, there must be Enquiry.cshtml
View Page in Home
Folder in Views.
2. Controller name and Views Folder name should be same.
3. Action Method and
Example:
.cshtml
file name should be same.In this example, I am going to add an Enquiry
Action Method in Home
Controller and then I will show you how you can create a View Pages for Enquiry
Action Method.
1. Open
HomeController.cs
and add following code.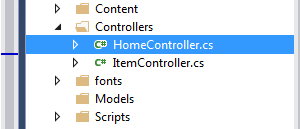
using System.Web.Mvc; namespace CompShop.Controllers { public class HomeController : Controller { public ActionResult Index() { return View(); } public ActionResult About() { ViewBag.Message = "Your application description page."; return View(); } public ActionResult Contact() { ViewBag.Message = "Your contact page."; return View(); } public ActionResult Enquiry() { return View(); } } }
2. Now, right click on
Enquiry()
Action Method and choose Add View.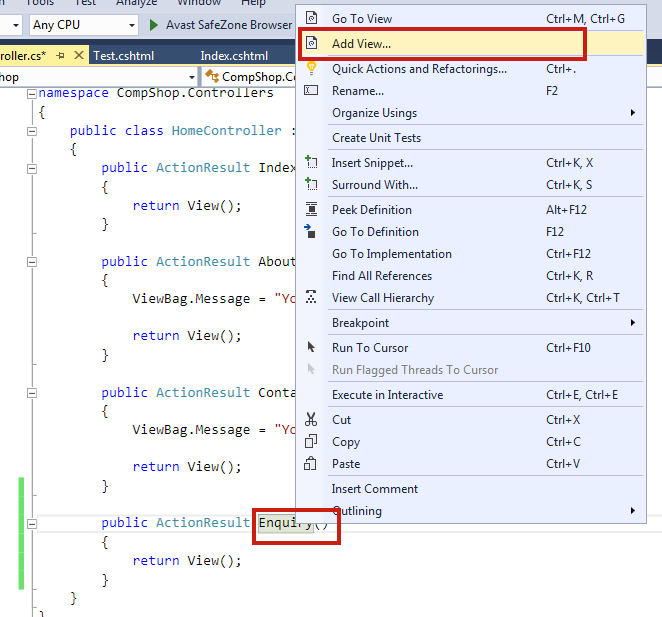
3. Add View Dialog box will Open. Don't change View name and it should be named as
Enquiry
. Select Empty (without model) in Template combo box and click Add.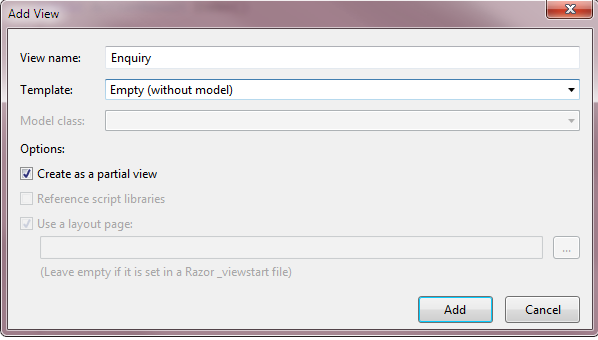
4. You will see that an
Enquiry.cshtml
is added in Home
View Folder.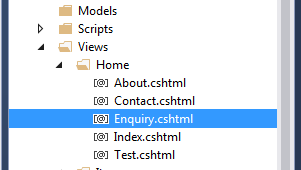
5. Open
Enquiry.cshtml
page and write following code in it.<h1>Enquiry Page</h1> <h2 style="color:purple">Welcome to Item List Enquiry Page.</h2>
6. Run your project and go to the following link.
http://localhost:1233/Home/Enquiry
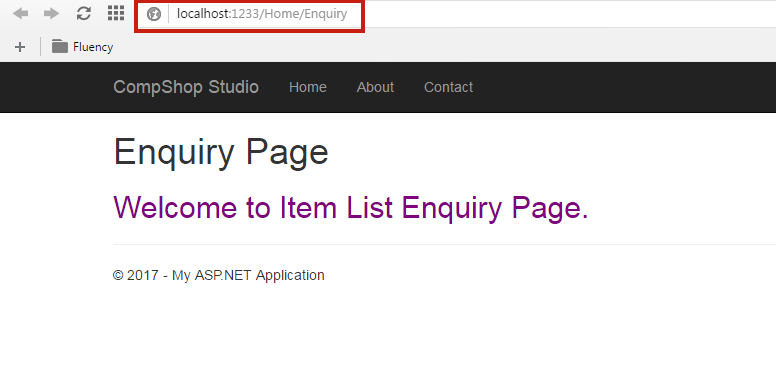
What If I add a View Page without Action Method
You will get HTTP: 404 Error. Let's understand it with an example.
1. Go to Solution Explorer Views Home.
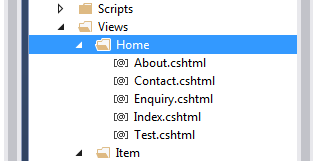
2. Right Click on Home Folder Add View.
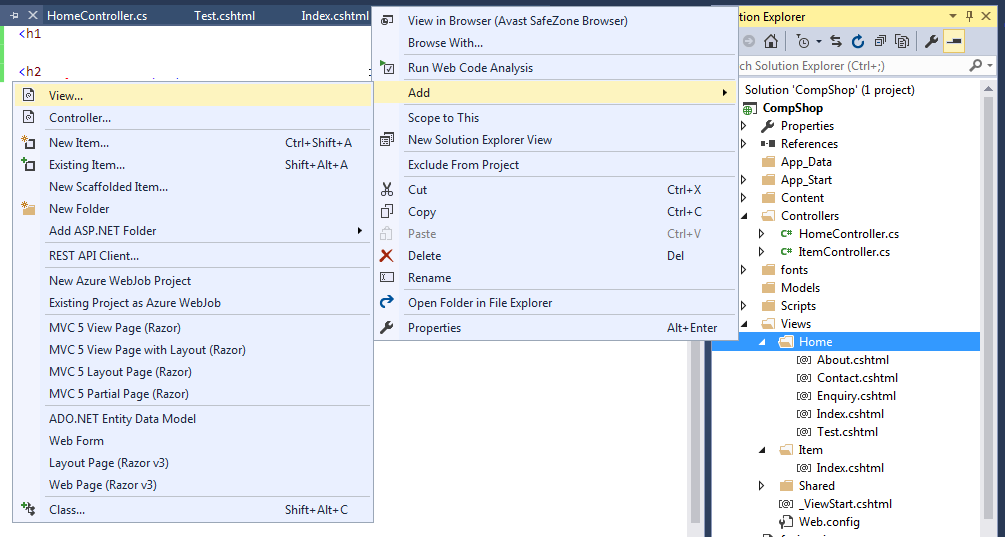
3. Give View Name: Purchase and click Add.
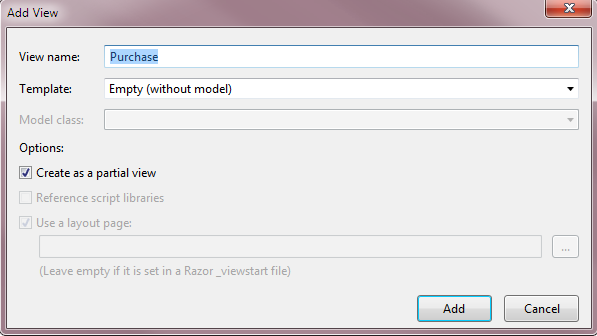
4. Open Purchase.cshtml and add following code in it.
Purchase Page
Welcome to Purchase Page.
5. Run your project and go to the following link.
http://localhost:1233/Home/Purchase
6. You will get HTTP 404 Error. Why? Because you haven't created an Action Method which renders these Purchase.cshtml to the client browser.
Output:
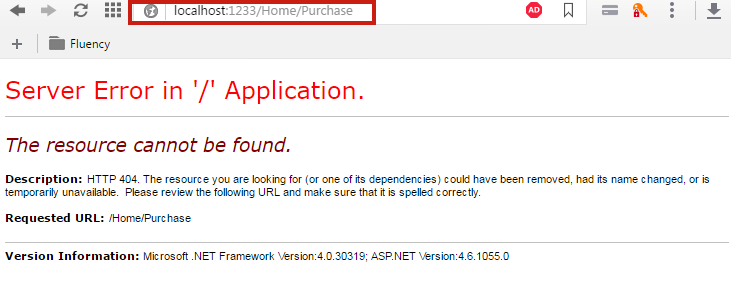
7. Go to Controllers HomeController.cs and add following code in it.
public ActionResult Purchase() { return View(); }
8. Now, run your project, you will see the Purchase Page instead of 404 Error page.
Output: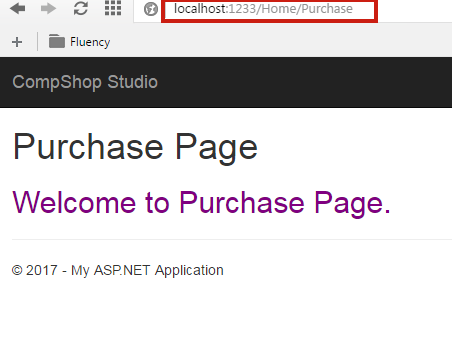
Summary
My motive is only to teach you how to add View Page in ASP.NET MVC 5 and making you understand how View page works with controllers and action method. I Hope, now it is clear to you but there would be several questions must be running on your mind like,
1. How to create Master Page Layout that gives consistent look to your site.2. How to apply custom CSS/HTML template to design your site.
3. There are lots of free CSS/HTML themes are available for free download and you want to apply these themes to your ASP.NET MVC5 websites.
Don't worry, in the next some chapter, you will learn how to design Master Page Layout in ASP.NET MVC5.