1. What is
Html.DropDownList
and Html.DropDownListFor
Methods?2. What is the difference between
Html.DropDownList
and Html.DropDownListFor
method?3. Html.DropDownList Example
4. Html.DropDownListFor Example
5. How Bind Html.DropDownListFor with a Model
6. How to display List item in DropDownList
7. How to send DropDownList Value to Controller
8. Html Attributes of Html.DropDownList
DropDownList is a visual control with down arrow which displays a list of items and forces you to choose only one item at a time. DropDownList is not editable, it means the user cannot enter own value and limited to choose an item from the selection.
Html.Helper
class provides two extension method for working with DropDownList.a. Html.DropDownList
b. Html.DropDownListFor
@Html.DropDownList: Definition and Example
Html.DropDownList
is a loosely type that means it is not strongly bound to any list items and model properties.
Html.DropDownList(string name, IEnumerable<SelectLestItem> selectList, string optionLabel, object htmlAttributes)
b. IEnumerable<SelectLestItem> selectList is the list of items
c. string optionLabel is the optional informative string like please choose item, select item, select value etc.
d. object HtmlAttributes adds extra features to dropdownlist.
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public TeaType SelectTeaType { get; set; } } public enum TeaType { Tea, Coffee, GreenTea, BlackTea } }
Index.cshtml
@using HtmlHelperDemo.Models @model UserModel <br /><br /> @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Select Tea Type: </b> @Html.DropDownList("SelectTeaType", new SelectList(Enum.GetValues(typeof(TeaType))),"--Select TeaType--") <input type="submit" value="submit" /> } <h4>You Selected</h4> <b>Tea Type: </b>@ViewBag.TeaType
HomeController.cs
using System.Web.Mvc; using HtmlHelperDemo.Models; namespace HtmlHelperDemo.Controllers { public class HomeController : Controller { public ActionResult Index() { return View(); } [HttpPost] public ActionResult Index(UserModel model) { var selectedValue = model.SelectTeaType; ViewBag.TeaType = selectedValue.ToString(); return View(); } } }
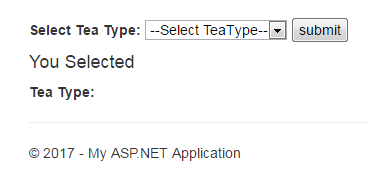
Html Output:
<select data-val="true" data-val-required="The SelectTeaType field is required." id="SelectTeaType" name="SelectTeaType"> <option value="">Select TeaType</option> <option>Tea</option> <option selected="selected">Coffee</option> <option>GreenTea</option> <option>BlackTea</option> </select>
You have noticed that 2 extra attributes are added in the html output: data-val and data-val-required.
Data-val ="true" and data-val-required="The SelectTeaType field is required." are coming because By default MVC adds [Required] attribute to non-nullable value types. To fix it, add the following line into Application_Start method in Global.asax file.
DataAnnotationsModelValidatorProvider.AddImplicitRequiredAttributeForValueTypes = false;
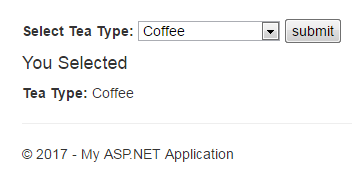
@Html.DropDownListFor: Definition and Example
Html.DropDownListFor is strongly bounded with model properties and checks for all errors at compile time. Before using this method, you must bind views with a model. Add model name in the top of the view as follows:
@using HtmlHelperDemo.Models @model UserModel
Define:
You can define
Html.DropDownListFor
as follows:
Html.DropDownListFor(model => model.property, IEnumerable<SelectLestItem> selectList, string optionLabel, object htmlAttributes)
b. IEnumerable<SelectLestItem> selectList: It is the list of items.
c. string optionLabel is the optional informative string like please choose item, select item, select value etc.
d. object HtmlAttributes adds extra features to dropdownlist.
Example:
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public TeaType SelectTeaType { get; set; } } public enum TeaType { Tea, Coffee, GreenTea, BlackTea } }
Index.cshtml
@using HtmlHelperDemo.Models @model UserModel <br /><br /> @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Select Tea Type: </b> @Html.DropDownListFor(m => m.SelectTeaType, new SelectList(Enum.GetValues(typeof(TeaType))), "--Select TeaType--") <input type="submit" value="submit" /> } <h4>You Selected</h4> <b>Tea Type: </b>@ViewBag.TeaType
HomeController.cs
using System.Web.Mvc; using HtmlHelperDemo.Models; namespace HtmlHelperDemo.Controllers { public class HomeController : Controller { public ActionResult Index() { return View(); } [HttpPost] public ActionResult Index(UserModel model) { var selectedValue = model.SelectTeaType; ViewBag.TeaType = selectedValue.ToString(); return View(); } } }
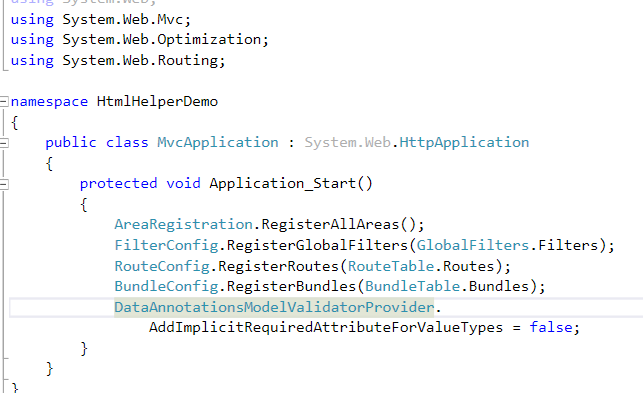
Html output
Summary
In this chapter, you learned basic Html.DropDownList
operation with complete programming example. Here I am adding some more programming examples on DropDownList, you must check and learn them for better understanding. In the next chapter, you will learn Html.ListBox.