In this chapter, you will learn:
1. What is ModelState Object?
2. How to validate input using Model.isValid Property?
3. How to display error message using ValidationMessageFor extension method.
1. What is ModelState Object?
2. How to validate input using Model.isValid Property?
3. How to display error message using ValidationMessageFor extension method.
ModelState is a property of controller that is used for validating form in server side. You must add System.Web.Mvc namespace in order to use it. ModelState is a collection of name and value pair that was submitted to the server during post. It also contains a collection of error messages for each value submitted.
Create Controllers and Methods
Step 1. Create a New Project or Start any existing ASP.NET MVC Project.
Step 2. Add a
StudentModel.cs
and paste following code.namespace FormValidation.Models { public class StudentModel { public string Name { get; set; } public int Age { get; set; } } }
Step 3. Add the following code in Index.cshtml page.
@{ ViewBag.Title = "Home Page - Student Details"; } @model FormValidation.Models.StudentModel <h2>Student Details</h2> @using (Html.BeginForm("StudentDetails", "Home", FormMethod.Post)) { <ol> <li> @Html.LabelFor(m => m.Name) @Html.TextBoxFor(m => m.Name) @Html.ValidationMessageFor(m => m.Name) </li> <li> @Html.LabelFor(m => m.Age) @Html.TextBoxFor(m => m.Age) @Html.ValidationMessageFor(m => m.Age) </li> </ol> <input type="submit" value="Save Student Details" /> } <h3 style="color:green">Student Details</h3> <h4 style="color:green"> <b>Name: @ViewBag.name<br /> <b>Age: @ViewBag.age<br /> </h4>
Steps 4. Add following action method in
HomeController.cs
.[HttpPost] public ActionResult StudentDetails(StudentModel sm) { if(string.IsNullOrEmpty(sm.Name)) { ModelState.AddModelError("Name", "Name Required"); } if (sm.Age == 0 || sm.Age > 120) { ModelState.AddModelError("Age", "Please Enter Valid Age between 1-120"); } if (ModelState.IsValid) { ViewBag.name = sm.Name; ViewBag.age = sm.Age; return View("Index"); } else { ViewBag.name = "No Data"; ViewBag.age = "No Data"; return View("Index"); } }
Step 5. Run this Project.
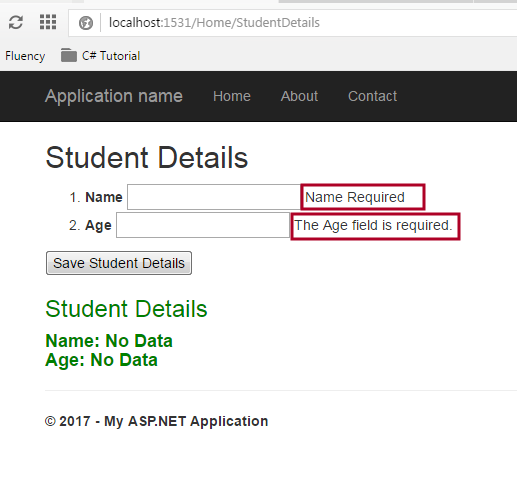
Let’s understand this ModelState Validation
1. Form is very simple and basic but you might notice that there is a new line added in form.
@Html.LabelFor(m => m.Name) @Html.TextBoxFor(m => m.Name) @Html.ValidationMessageFor(m => m.Name)
@Html.ValidationMessageFor
control display the error message for Name textbox. This error message sent from controller.
if(string.IsNullOrEmpty(sm.Name)) { ModelState.AddModelError("Name", "Name Required"); }
2. ModelState.AddModelError("Name", "Name Required");
- ModelState takes 2 parameter. First Parameter “Name” is a name of input control and 2nd parameter is a string error message.
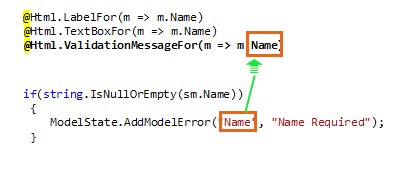
Summary
In this chapter, you learned how to use ModelState.isValid
property to examine user input at server side. In the next chapter, you will learn Validation using Data Annotation in MVC.