In this chapter, you will learn:
- What is
Html.Display
andHtml.DisplayFor
- How to define
Html.Display
Helper Method - How to Bind
Html.DisplayFor
to a Model - How to Display Textbox string into Html.Display Method
- Programming Example of Html.DisplayFor
What is Html.Display and Html.DisplayFor Method?
Html.Display
and Html.DisplayFor both are used to print string value to the screen. However, you can also use plain HTML tag for displaying text on the screen but Html.Display Extension Method provides more formatting option. Html.Display is loosely typed method whereas Html.DisplayFor is strongly typed method.
Programming Example
Model: UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public string UserName { get; set; } } }
View: Index.cshtml
@using HtmlHelperDemo.Models @model UserModel <h1>Html.DisplayFor Example</h1> @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <span>Enter Your Name:</span> @Html.TextBoxFor(m => m.UserName) <input id = "Submit" type = "submit" value = "submit" /> } <hr /> <strong>Strongly Typed Value:</strong> @Html.DisplayFor(m => m.UserName)<br /> <strong>Loosely Typed Value: </strong> @Html.Display("UserName")
Controller: HomeController.cs
using System.Web.Mvc; using HtmlHelperDemo.Models; namespace HtmlHelperDemo.Controllers { public class HomeController : Controller { public ActionResult Index() { return View(); } [HttpPost] public ActionResult Index(FormCollection fc, UserModel um) { if (ModelState.IsValid) { um.UserName = fc["UserName"]; return View(um); } else { return View(); } } } }
Output
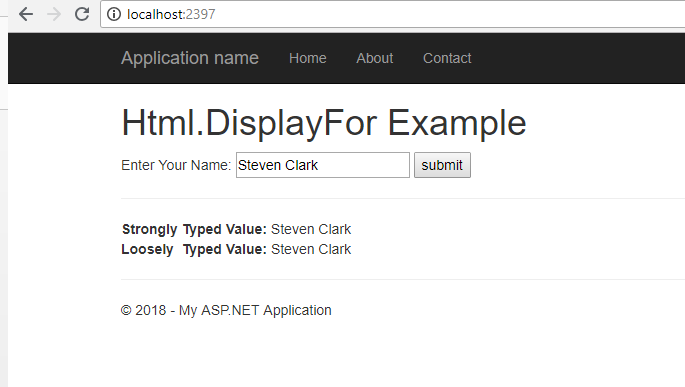