1. What is Scaffolding in ASP.NET MVC 5?
2. What is the use of Scaffolding?
3. How to add Scaffolded Item in project?
What is Scaffolding in ASP.NET MVC 5?
Scaffolding
is a code generation framework that automatically adds codes and creates view pages and controllers for your project. Scaffolding makes developer job easier by creating controllers and view pages for the data model. It generates codes and pages for CRUD(Create, Read, Update and Delete) Operation. In this chapter, I will show you how to use Scaffolding in your project. It is a complete programming example and contains step by step guide so if you are new in MVC then absolutely don’t worry and start reading this chapter.
How to Use Scaffolding in ASP.NET MVC 5?
ScaffoldProject
. ItemListModel
. Right click on Model Add Class. Give class name as ItemListModel.cs
and click Add button.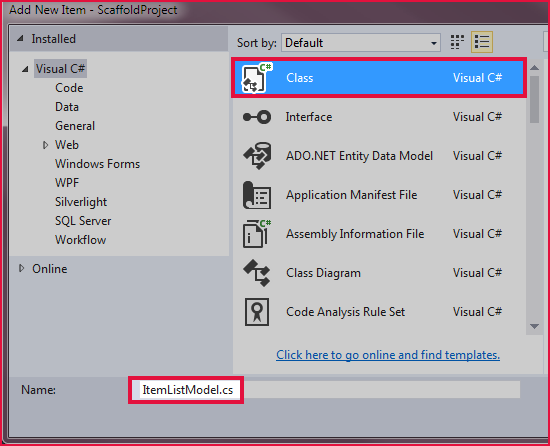
ItemListModel
class.using System.Data.Entity; namespace ScaffoldProject.Models { public class ItemListModel { public int ID { get; set; } public string Name { get; set; } public string Category { get; set; } public decimal Price { get; set; } public class ItemListContext : DbContext { public DbSet<ItemListModel> itemListModel { get; set; } } } }
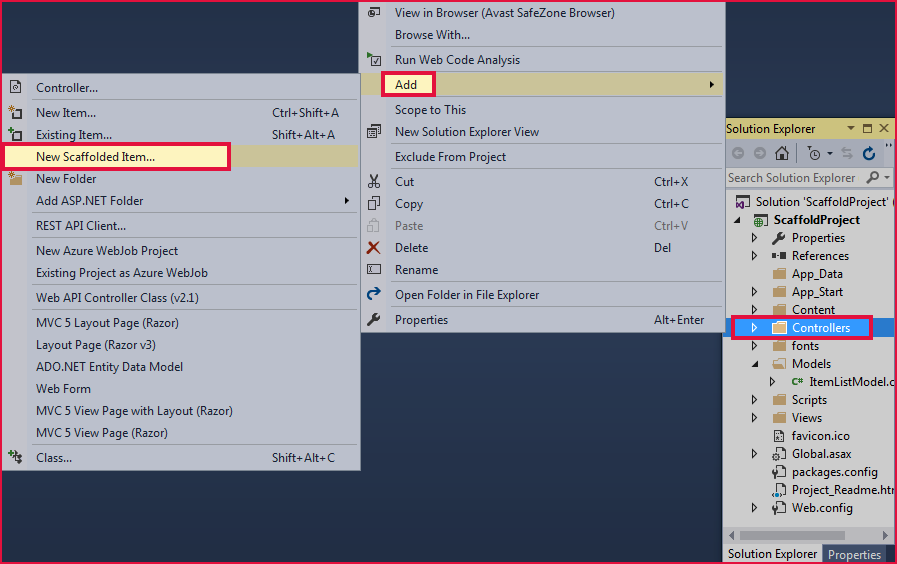
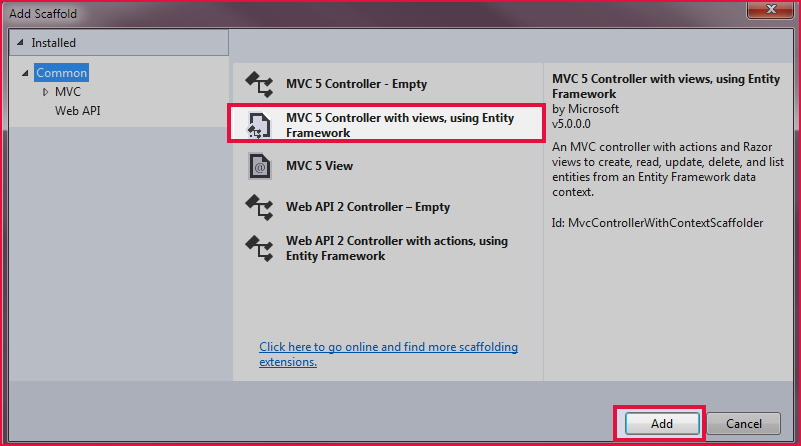
ItemListModel (ScaffoldProject.Models)
b. Data Context class: Create a new data context class by clicking on +
button.
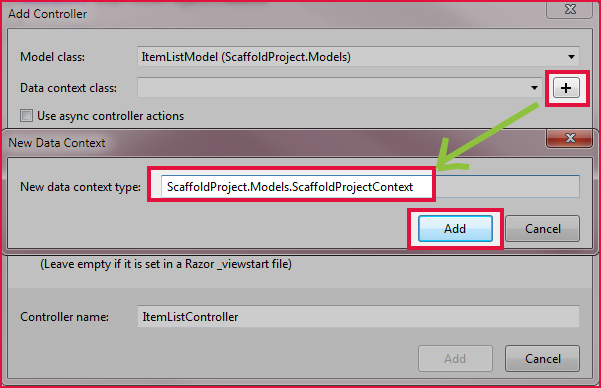
c. Set Controller name: ItemListController
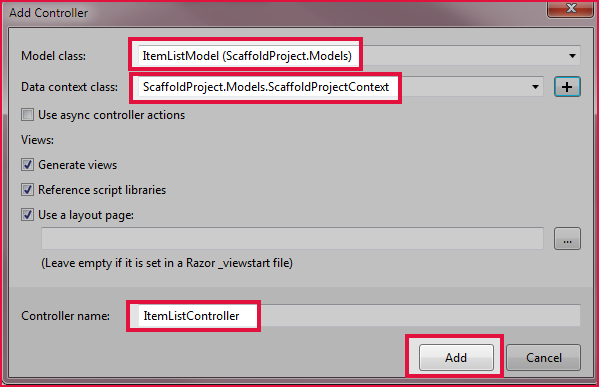
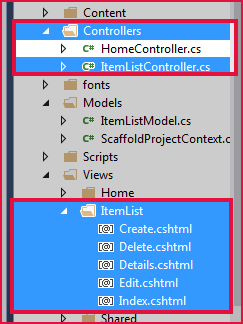
Navigate to following link: http://localhost:3083/ItemList/
Output Images: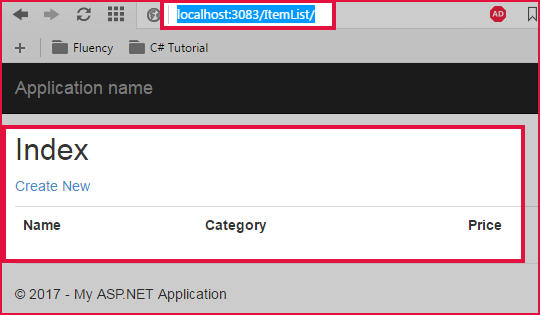
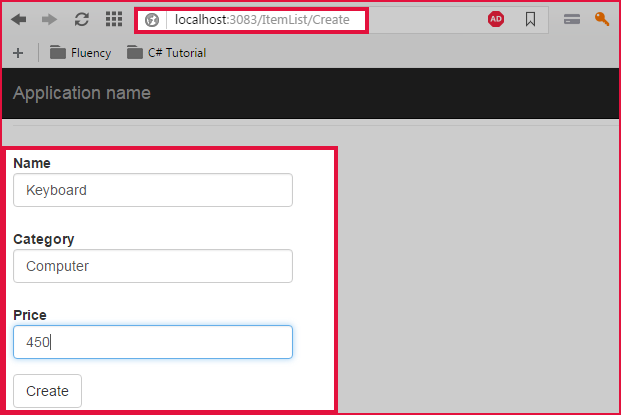
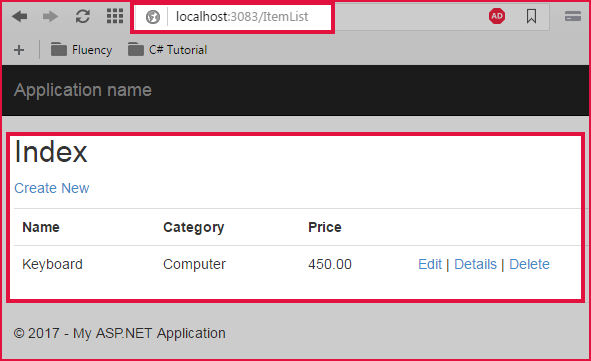
Summary
In this chapter, you learned what Scaffolded item is in the ASP.NET MVC 5 and how to use it in the program. Scaffolded makes developer job easier and creates controllers and views automatically based on models and database tables. In the next chapter, you will learn Model Binding in ASP.NET MVC 5.