In this tutorial, you will learn:
- What is
Html.ValidationMessageFor
in ASP.Net MVC5? - How to define
Html.ValidationMessageFor
? - Programming Example
Html.ValidationMessageFor
is a strongly typed method that is used for display error message for input control if the invalid value entered. You can define Html.ValidationMessageFor as follows:
@Html.ValidationMessageFor(Model Property, "String Message", Html Attribute })
Model Property binds the ValidationMessageFor helper method to a model property.
String Message is used for displaying a custom error message
Html Attribute allows you to insert CSS.
Mostly there are 3 parts of validating a control and displaying a message.
- Create Rules for model properties in Model class.
- Validating in Controller
- Displaying an Error message in View.
1. Create Rules for model properties in Model class.
using System.ComponentModel.DataAnnotations; namespace HtmlHelperDemo.Models { public class UserModel { [Required(ErrorMessage = "Name is Required. It cannot be empty")] public string UserName { get; set; } } }
2. Validating in Controller
[HttpPost] public ActionResult Index(UserModel um) { if (ModelState.IsValid) { return View(um); } else { return View(); } }
3. Displaying an Error message in View.
@using HtmlHelperDemo.Models @model UserModel <h3><code>Html.ValidationMessageFor Example</code></h3> <br /> @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <strong>Name </strong> @Html.EditorFor(m => m.UserName) @Html.ValidationMessageFor(m => m.UserName, "", new { @class = "text-danger" }) <br /><br /> <input id="Submit" type="submit" value="submit" /> } <br /><br /> <strong>User Name:</strong> @Html.DisplayFor(m => m.UserName)<br />
Html Output
<input class="input-validation-error text-box single-line" data-val="true" data-val-required="Name is Required. It cannot be empty" id="UserName" name="UserName" type="text" value="" /> <span class="field-validation-error text-danger" data-valmsg-for="UserName" data-valmsg-replace="true">Name is Required. It cannot be empty </span>
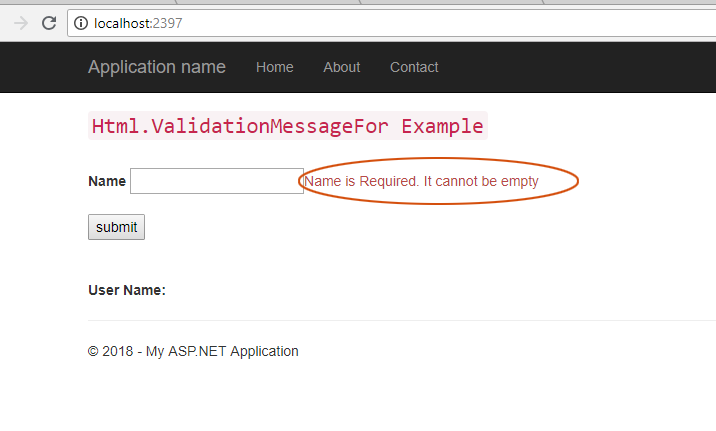
In this tutorial, I tried to give you a fundamental knowledge about What is and How to Use Html.ValidationMessageFor in ASP.Net MVC 5. You can learn complete Html Form Validation with an example in ASP.Net MVC 5 here.
Data Annotation Validation With Example In ASP.NET MVC