1. What is Model Binding in MVC?
2. How Model Binding Works
3. Programming Example
What is Model Binding in MVC?
Do you ever wondered that how the form data gets automatically bound with model properties? Let’s see the picture below:
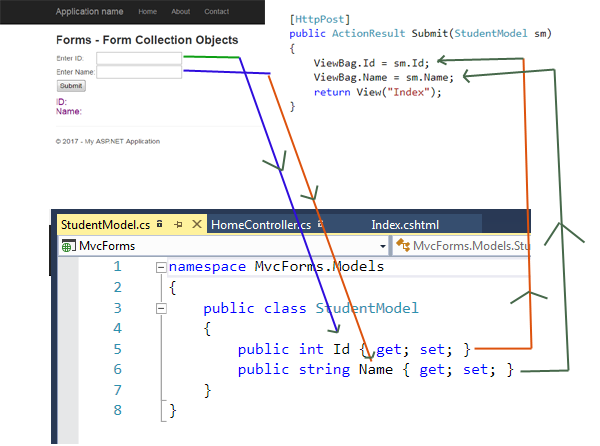
You have noticed the following thing:
1. It is much cleaner code and there is no fancy coding.
2. No type casting needed.
3. No need to remember model properties because it appears in IntelliSense.
4. Adding and deleting properties from model is easy.
Model binding makes all these tasks easy. Simply, Model binding means bind your input control to the respective model properties and once it is bound, enjoy the power and simplicity of ASP.NET MVC. Model binding maps incoming data to corresponding model properties.
In this tutorial, I will discuss several model binding techniques that will help you in access input data in models and controllers.
2. Simple Binding
3. Class Binding
4. Complex Binding
5. FormCollection Binding
6. Bind Attribute
No Binding
No Binding means access form data directly without binding form to a specific model.
Example:namespace MvcForms.Models { public class StudentModel { public int Id { get; set; } public string Name { get; set; } } }
<h2>Mvc Forms - Model Binding</h2> @using (Html.BeginForm()) { <table> <tr> <td>Enter ID: </td> <td>@Html.TextBox("Id")</td> </tr> <tr> <td>Enter Name: </td> <td>@Html.TextBox("Name")</td> </tr> <tr> <td colspan="2"><input type="submit" value="Submit"></td> </tr> </table> } <h4 style="color:purple"> ID: @ViewBag.Id<br /> Name: @ViewBag.Name<br /> </h4>
public ActionResult Index() { if (Request.Form.Count > 0) { ViewBag.Id = Request.Form["Id"]; ViewBag.Name = Request.Form["Name"]; return View("Index"); } return View(); }
2. Simple Binding
In simple binding, pass the parameter in action method with the same name as model properties and MVC Default Binder will automatically map correct action method for the incoming request.
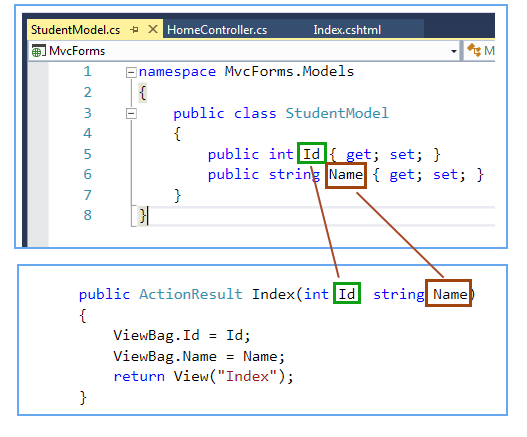
public ActionResult Index(int Id, string Name) { ViewBag.Id = Id; ViewBag.Name = Name; return View("Index"); }
3. Class Binding
In Class Binding, pass model as a parameter in action method and then access its entire member variable.
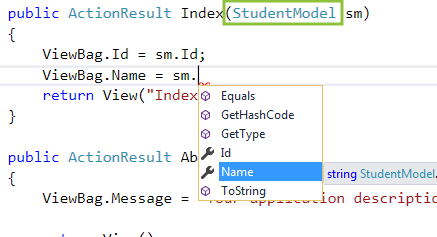
public ActionResult Index(StudentModel sm) { ViewBag.Id = sm.Id; ViewBag.Name = sm.Name; return View("Index"); }
4. Complex Binding
Complex Binding means apply multiple related models in a single view. Here, in this example, I will show you how to bind multiple models in a single view.
namespace MvcForms.Models { public class StudentModel { public int Id { get; set; } public string Name { get; set; } public CourseModel courseModel { get; set; } } }
namespace MvcForms.Models { public class CourseModel { public int Id { get; set; } public string Course { get; set; } public string Duration { get; set; } } }
I have added CourseModel
as a property in StudentModel
, so the StudentModel
has right to access CourseModel
Property.
@model MvcForms.Models.StudentModel <h2>Mvc Forms - Model Binding</h2> @using (Html.BeginForm()) { <table> <tr> <td>Enter ID: </td> <td>@Html.TextBoxFor(m => m.Id)</td> </tr> <tr> <td>Enter Name: </td> <td>@Html.TextBoxFor(m => m.Name)</td> </tr> <tr> <td>Enter Course: </td> <td>@Html.TextBoxFor(m => m.courseModel.Course)</td> </tr> <tr> <td>Enter Duration: </td> <td>@Html.TextBoxFor(m => m.courseModel.Duration)</td> </tr> <tr> <td colspan="2"><input type="submit" value="Submit"></td> </tr> </table> } <h4 style="color:purple"> ID: @ViewBag.Id<br /> Name: @ViewBag.Name<br /> Course: @ViewBag.Course<br /> Duration: @ViewBag.Duration<br /> </h4>
[HttpGet] public ActionResult Index() { return View(); } [HttpPost] public ActionResult Index(StudentModel sm) { ViewBag.Id = sm.Id; ViewBag.Name = sm.Name; ViewBag.Course = sm.courseModel.Course; ViewBag.Duration = sm.courseModel.Duration; return View("Index"); }
Output:
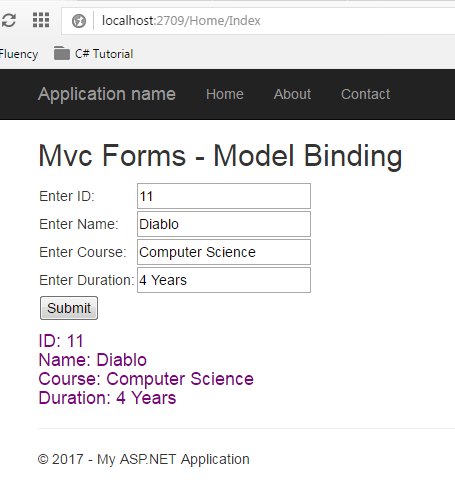
Summary
In this chapter, I discussed model binding in MVC with the help of an easy and simple example. However, there are also various ways by which you can bind views with models and controllers. Just read this chapter, If you are not comfortable in transferring data between models and views.