1. What is Html.TextBox and Html.TextBoxFor Helper Class?
2. What is the difference between Html.TextBox and Html.TextBoxFor method?
3. Html.TextBox Example
4. Html.TextBoxFor Example
5. How Bind Html.TextBoxFor with a Model
6. How to send data from TextBox to Controller
7. Html Attributes of Html.TextBox
@Html.TextBox()
Html.TextBox
is extension method of HtmlHelper class that draws HTML TextBox and accept input from user. Html.TextBox is loosely typed method that renders plain textbox input and doesn't get bounded with any model properties. Because it is loosely type bounded, it also does not check for error at compile time so, if you passed wrong string as parameter that don’t match to model properties, you are likely to get runtime error.
You can define Html.TextBox()
method as below:
Html.TextBox(string name, string value, object htmlAttributes)
a. string name is the name of the TextBox.
b. string value is the text, appears inside TextBox
c. object htmlAttributes is the place where you can put your own css properties and class.
Programming Example of
Html.TextBox(
) method in ASP.NET MVC
Model:
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public string Name { get; set; } } }
View:
Index.cshtml
@using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Name: </b> @Html.TextBox("Name","Enter Name", new { style="color:red" }) }
Html Output
<input id="Name" name="Name" style="color:red" type="text" value="Enter Name" />
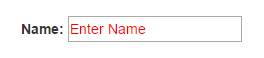
@Html.TextBoxFor()
Html.TextBoxFor
also used for drawing text box in asp.net web pages but it is strongly bounded with model properties. The benefit of using Html.TextBoxFor is, it checks for error at compile time and saves you from any uncertain runtime error. Because it is strongly bounded with model properties, you will also get Intellisense suggestion when using it.
You can define @Html.TextBoxFor()
as follow:
TextBoxFor(model => model.properties, object htmlAttributes)
a. model uses the model class that view is bounded.
b. htmlAttributes defines space for css properties and class.
In order to bind Html.TextBoxFor()
with a model, you must define model in view page.
@model HtmlHelperDemo.Models.UserModel
Programming Example of Html.TextBoxFor() method in ASP.NET MVC
Model:
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public string Name { get; set; } } }
View:
Index.csthml
@model HtmlHelperDemo.Models.UserModel @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { @Html.TextBoxFor(m => m.Name, new {@Value = "Enter Name", @class = "red" }) } <style> .red { color:red; } </style>
Html Output
<input Value="Enter Name" class="red" id="Name" name="Name" type="text" value="" />
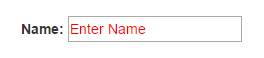
How to Send Data from Html.TextBoxFor to controller
It is very simple of getting TextBoxFor data in the controller. Here, is an example.
[HttpPost] public ActionResult Index(UserModel u) { ViewBag.Name = u.Name; return View(); }
HTML Attributes
Html Attributes set Html Properties for input control like width, color, value, css class etc. Attributes provides additional information about elements and always comes with name="value" pair.
Example:@Html.TextBoxFor(m => m.Name, new { @Value = "Enter Name", @class = "red", @disabled = "true", })
Here is the list of Html Attributes
Difference Between Html.TextBox and Htm.TextBoxFor method.
Html.TextBox() | Html.TextBoxFor() |
---|---|
It is loosely typed. It may be or not bounded with Model Properties. | It is strongly typed. Means, It will be always bounded with a model properties. |
It requires property name as string. | It requires property name as lambda expression. |
It doesn’t give you compile time error if you have passed incorrect string as parameter that does not belong to model properties. | It checks controls at compile time and if any error found it raises error. |
It throws run time error. Run time error gives bad impression to user and if the project is worthy, you may lose your client simply because of one error. | It throws compile time error which can be corrected before launching the project. It enhances user experience without throwing error. |
Summary:
In this chapter, you learned Html.TextBox() and Html.TextBoxFor() Helper Extension method in ASP.NET MVC 5 with complete programming example. I kept this chapter simple, short and easy so hopefully you will not get any problem in understanding this chapter. In the next chapter, you will learn about Html.TextArea() extension method.