In this chapter, you will learn:
1. What is FormCollection Object
2. Retrieving Form Data using FormCollection in MVC 5
3. FormCollection Object Programming Example
1. What is FormCollection Object
2. Retrieving Form Data using FormCollection in MVC 5
3. FormCollection Object Programming Example
What is FormCollection Object?
A FormCollection Object is used to retrieve form input values in action method. In the previous chapter, I have explained 4 different ways to create form and gather form data in action method. FormCollection object makes programmers job easier and forms data can be easily accessed in action method.
FormCollection Example - Retrieving Form Data
Step 1: Create a New ASP.NET MVC Project or open Existing Project.
Step 2: Create a new Model
StudentModel.cs
and add the following code in it.
namespace MvcForms.Models { public class StudentModel { public int Id { get; set; } public string Name { get; set; } public bool Addon { get; set; } } }
Step 3: Go to Views Home
Index.cshtml
and add the following code to create form.
<h3><b>Forms - Form Collection Objects</b></h3> @using (Html.BeginForm("Submit", "Home", FormMethod.Post)) { <table> <tr> <td>Enter ID: </td> <td>@Html.TextBox("Id")</td> </tr> <tr> <td>Enter Name: </td> <td>@Html.TextBox("Name")</td> </tr> <tr> <td>Addon: </td> <td>@Html.CheckBox("Addon", false)</td> </tr> <tr> <td colspan="2"><input type="submit" value="Submit"></td> </tr> </table> } <h4 style="color:purple"> ID: @ViewBag.Id<br /> Name: @ViewBag.Name<br /> Addon: @ViewBag.Addon </h4>
Step 4: Create following action method in HomeController
[HttpPost] public ActionResult Submit(FormCollection fc) { ViewBag.Id = fc["Id"]; ViewBag.Name = fc["Name"]; bool chk = Convert.ToBoolean(fc["Addon"].Split(',')[0]); ViewBag.Addon = chk; return View("Index"); }
Step 5: Run your Project.
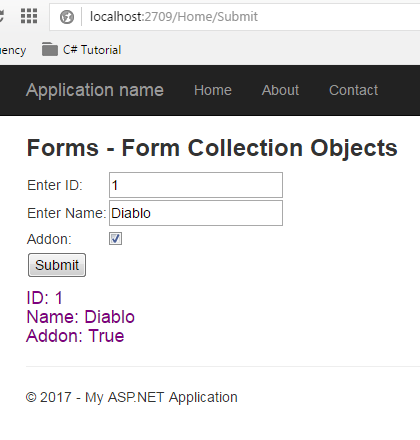
Let's understand this code
1. Created simple MVC Forms using HTML Helper
2. In Submit Action Method, I have initialized FormCollection object
fc
.3. FormCollection value can be accessed either giving input control
"id"
or index number.4. If you will not split Boolean value then you will get
"true, false"
both output, that's why I have gathered checkbox value in Boolean type variable and split them.bool chk = Convert.ToBoolean(fc["Addon"].Split(',')[0]);
Summary
In this chapter, I tried to teach you FormCollection object with an easy and complete example. In the next chapter, you will learn Form Validation Techniques in ASP.NET MVC.