In this tutorial, you will learn:
- What is the use of ValidationSummary in ASP.NET MVC5?
- How to use ValidationSummary in MVC?
- Programming Example
Html.ValidationSummary
is used to display error message at a fixed and only place. It summarizes all the error, collects them and show them in one place after clicking the submit button. Instead of displaying message next to each control, it is a good practice to show summarize error in a single place using Html.ValidationSummary
method in ASP.NET MVC 5 C#.
Programming Exmaple
Model:
UserModel.cs
- using System.ComponentModel.DataAnnotations;
- namespace HtmlHelperDemo.Models
- {
- public class UserModel
- {
- [Required(ErrorMessage="UserName is Required.")]
- public string UserName { get; set; }
- [Required(ErrorMessage ="Mobile Number is Required")]
- public string MobileNumber { get; set; }
- }
- }
Controller:
HomeController.cs
- using System.Web.Mvc;
- using HtmlHelperDemo.Models;
- namespace HtmlHelperDemo.Controllers
- {
- public class HomeController : Controller
- {
- public ActionResult Index()
- {
- return View();
- }
- [HttpPost]
- public ActionResult Index(UserModel user)
- {
- return View();
- }
- }
- }
View:
Index.cshtml
- @model HtmlHelperDemo.Models.UserModel
- @{
- ViewBag.Title = "Home Page";
- }
- <div class="jumbotron">
- <h1>User Entry Form</h1>
- </div>
- <div class="row">
- @using (Html.BeginForm("Index", "Home", FormMethod.Post))
- {
- <table>
- <tr>
- <td>User Name : </td>
- <td>@Html.TextBoxFor(m => m.UserName)</td>
- </tr>
- <tr>
- <td>Mobile No : </td>
- <td>@Html.TextBoxFor(m => m.MobileNumber)</td>
- </tr>
- <tr>
- <td colspan="2"><input type="submit" value="submit" /></td>
- </tr>
- </table>
- @Html.ValidationSummary(false, "", new { @class = "alert-danger"})
- }
- @Scripts.Render("~/bundles/jquery")
- @Scripts.Render("~/bundles/jqueryval")
- </div>
Output
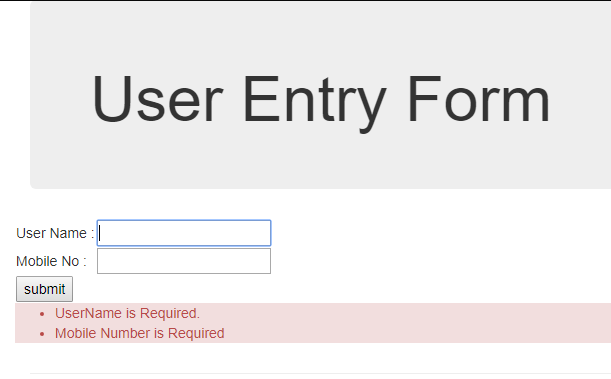
This tutorial explains about Html.ValidationSummary
control in ASP.NET MVC. Using the complete programming example, you will be able to understand this topic more clearly. In the next chapter you will learn Encoding in MVC.