1. What is Html.TextArea and Html.TextAreaFor Helper Class?
2. What is the difference between Html.TextArea and Html.TextAreaFor method?
3. Html.TextArea Example
4. Html.TextAreaFor Example
5. How Bind Html.TextAreaFor with a Model
6. How to send data from TextArea to Controller
7. Html Attributes of Html.TextArea
TextArea is a multiline text input control that can accept a large number of string lines. Usually, size of TextArea is divided into rows and column and you can set it according to your needs.
@Html.TextArea()
Html.TextArea
is loosely typed text input control that takes string as parameter. This string may be or not same as model property. Because it doesn't bind string parameter with model property and not check for error at compile time so there can be a huge chance of getting a runtime error.
There are various overload method in Html.TextArea()
input control but commonly you can define as like:
Html.TextArea(string name, string value, object htmlAttributes)
Html.TextArea() Example
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public string Name { get; set; } } }
Index.cshtml
@using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Name: </b>@Html.TextArea("Name","Enter Name", new { @style="color:red" }) }
Html Output
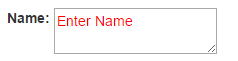
@Html.TextAreaFor()
Html.TextAreaFor()
strongly bounded with Models properties. It checks input control at compile time and saves you from uncertain runtime errors.
@model HtmlHelperDemo.Models.UserModel
2. Use lambda expression for accessing this model properties.
@Html.TextAreaFor(m => m.Name)
@Html.TextAreaFor()
Example
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public string Name { get; set; } } }
Index.cshtml
@model HtmlHelperDemo.Models.UserModel @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Name: </b>@Html.TextAreaFor(m => m.Name, new { @style = "color:red" }) }
Html Output
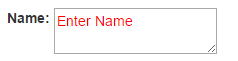
Html Attributes
Html Attributes set Html Properties for input control like width, color, value, CSS class etc. Attributes provide additional information about elements and always comes with name="value" pair.
Here is the list of Html Attributes
Example:@Html.TextAreaFor(m => m.Name, new { @value = "Enter Name", @class = "red", //@disabled="true", cols="50", rows="10", @title="Please Enter Your Name", @tabindex="0", //@accesskey="z", @align="left", @autofocus="true", @style="background-color:yellow; font-weight:bold", @draggable="true", //@hidden="true", //@maxlength="12", @minlength="5", @required="true" })
Html Output
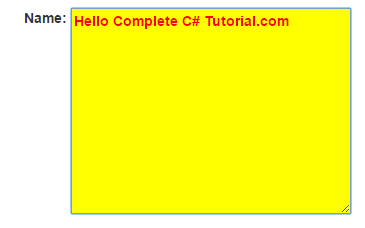
Difference between Html.TextArea()
and Html.TextAreaFor()
Extension Method
Html.TextArea() | Html.TextAreaFor() |
---|---|
It is loosely typed. It may be or not bounded with Model Properties. | It is strongly typed. Means, It will be always bounded with a model properties. |
It requires property name as string. | It requires property name as lambda expression. |
It doesn’t give you compile time error if you have passed incorrect string as parameter that does not belong to model properties. | It checks controls at compile time and if any error found it raises error. |
It throws run time error. Run time error gives bad impression to user and if the project is worthy, you may lose your client simply because of one error. | It throws compile time error which can be corrected before launching the project. It enhances user experience without throwing error. |
Summary:
In this chapter, you learned Html.TextArea()
and Html.TextAreaFor
Helper Extension method in ASP.NET MVC 5 with complete programming example. I kept this chapter simple, short and easy so hopefully you will not get any problem in understanding this chapter. In the next chapter, you will learn about Html.TextArea() extension method.