1. What is ViewBag, ViewData and TempData in ASP.NET MVC?
2. How to use ViewBag, ViewData and TempData for passing data from model to views?
In the previous chapter, you learned what is ViewModel and how to use it for sending model data to views. In this chapter, you will learn how to use ViewBag, ViewData and TempData for passing information from controllers/model to views.
Create Models and Controllers
Before Starting this section, you must create following model classes and controllers. If you have already created it in the previous chapter then skip creating these models and controllers.
Using System; namespace CompShop.Models { public class ItemModel { public int ID { get; set; } public string Name { get; set; } public string Category { get; set; } public decimal Price { get; set; } } }
BuyersCommentsModel – It retrieves Comments
using System; namespace CompShop.Models { public class BuyersCommentsModel { public int BuyersID { get; set; } public string BuyersName { get; set; } public string StarRating { get; set; } public string CommentsTitle { get; set; } public string Comments { get; set; } public DateTime Date { get; set; } } }
ItemCommentsController – It will retrieve Items and Comments from models. I have added some items and comments in controller class in order to reduce complexity. Using the same method you can display database value to view pages.
using System; using System.Collections.Generic; using System.Web.Mvc; using CompShop.Models; namespace CompShop.Controllers { public class ItemCommentsController : Controller { // GET: ItemComments public ActionResult Index() { return View(); } public ItemModel GetItemDetails() { ItemModel iModel = new ItemModel() { ID = 1, Name = "HP Printer", Category = "ComputerParts", Price = 8840 }; return iModel; } public List<BuyersCommentsModel> GetCommentList() { List<BuyersCommentsModel> CommentsList = new List<BuyersCommentsModel>(); CommentsList.Add(new BuyersCommentsModel() { BuyersID = 1, BuyersName = "John", StarRating = "4", CommentsTitle = "Nice Product", Comments = "I Purchased it 1 week ago and it is working fine.", Date = Convert.ToDateTime("27 August 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=2, BuyersName="Nicki", StarRating="2", CommentsTitle="Worst Product", Comments="Worst Product. Don't Buy It. I got damaged one.", Date=Convert.ToDateTime("12 June 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=3, BuyersName="Serena", StarRating="3.5", CommentsTitle="Satisfactory", Comments="Go for it. It does the same job and have the less price", Date=Convert.ToDateTime("18 March 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=4, BuyersName="William", StarRating="4.5", CommentsTitle="Superrr!!!", Comments="Don't think and buy it with confidence.", Date=Convert.ToDateTime("11 November 16") }); return CommentsList; } public ActionResult ItemCommentDisplay() { } } }
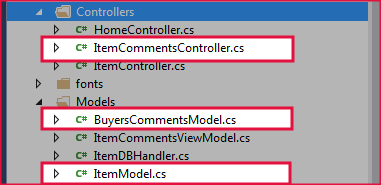
1. ViewBag
ViewBag is a dictionary of objects and it is used for passing data from controllers to view. It stores values in a dynamic string property and you need to also use this string to retrieve values in the view page. ViewBag is used for only one-time request and once it populates the value to views it becomes null. If any redirection occurs, then also ViewBag value becomes null. There is no typecasting needed in ViewBag.
ItemCommentsController.cs
and add the following highlighted action method.public ActionResult ItemCommentDisplay() { ViewBag.Item = GetItemDetails(); ViewBag.Comments = GetCommentList(); return View(); }
ItemCommentDisplay()
Action Method and create a View.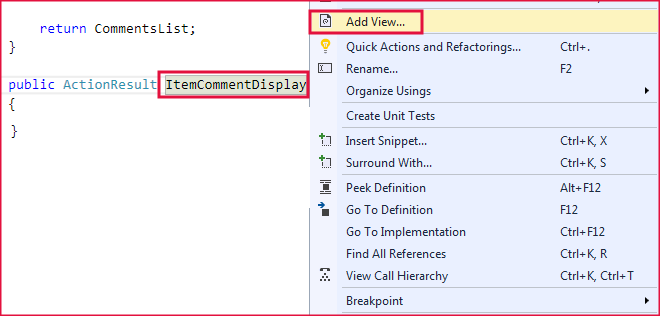
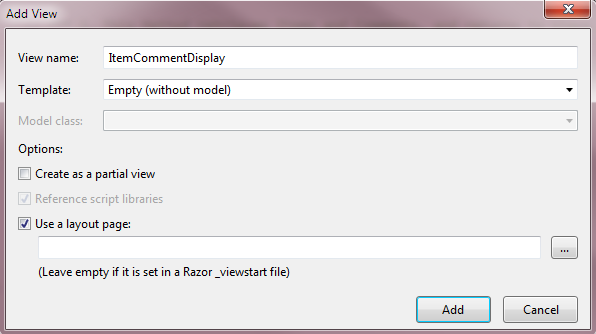
ItemCommentDisplay.cshtml
View Page.@using CompShop.Models @{ ItemModel Item = ViewBag.Item as ItemModel; List<BuyersCommentsModel> Comments = ViewBag.Comments as List<BuyersCommentsModel>; } <div> <h4><b>Pass Data using ViewBag</b></h4> <hr /> <dl class="dl-horizontal"> </dl> </div> <p> <!-- ITEM DETAILS --> <div style="background-color:burlywood; padding:5px;"> <h1 style="color:green">@Item.Name</h1> <b>Category:</b> @Item.Category<br /> <b>Price:</b> @Item.Price <hr /> </div> <!-- LOADING COMMENTS --> <h2>Comments</h2> @foreach (var comments in Comments) { <h4><b>@comments.CommentsTitle</b></h4> <b>@comments.BuyersName</b><br /> <b>Date:</b> @comments.Date <span>|</span> <b>Star Rating: </b>@comments.StarRating<span>/5</span> <br /> <i>@comments.Comments</i> <hr /> } </p>
Output:
http://localhost:1233/ItemComments/ItemCommentDisplay
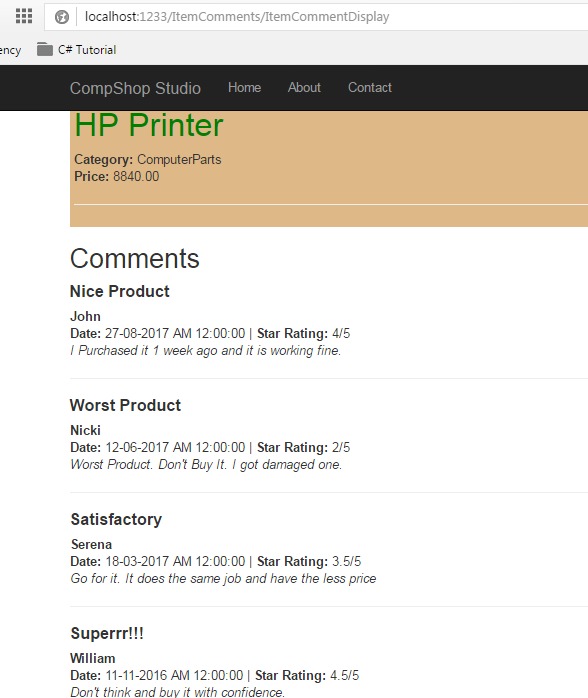
ViewData
ViewData is almost same as ViewBag and it is also used for passing models/controllers data to view. It stores data in Key-Value pair and it is also available for current request only. Means, if redirection occurs then ViewData value becomes null. You must check for null and typecast complex data type for avoiding errors.
ItemCommentsController.cs
and add the following highlighted action method.public ActionResult ItemCommentDisplay() { ViewData["Item"] = GetItemDetails(); ViewData["Comments"] = GetCommentList(); return View(); }
ItemCommentsController.cs
and change the ItemCommentDisplay()
action method.@using CompShop.Models @{ ItemModel Item = ViewData["Item"] as ItemModel; List<BuyersCommentsModel> Comments = ViewData["Comments"] as List<BuyersCommentsModel>; } <div> <h4><b>Pass Data using ViewBag</b></h4> <hr /> <dl class="dl-horizontal"> </dl> </div> <p> <!-- ITEM DETAILS --> <div style="background-color:burlywood; padding:5px;"> @if (Item != null) { <h1 style="color:green">@Item.Name</h1> <b>Category:</b> @Item.Category<br /> <b>Price:</b> @Item.Price } else { <h3 style="color:red">Sorry! Item Not Found</h3> } <hr /> </div> <!-- LOADING COMMENTS --> <h2>Comments</h2> @if (Comments != null) { foreach (var comments in Comments) { <h4><b>@comments.CommentsTitle</b></h4> <b>@comments.BuyersName</b><br /> <b>Date:</b> @comments.Date <span>|</span> <b>Star Rating: </b>@comments.StarRating<span>/5</span> <br /> <i>@comments.Comments</i> <hr /> } } else { <h3 style="color:red">No Comments Found!</h3> } </p>
Output
http://localhost:1233/ItemComments/ItemCommentDisplay
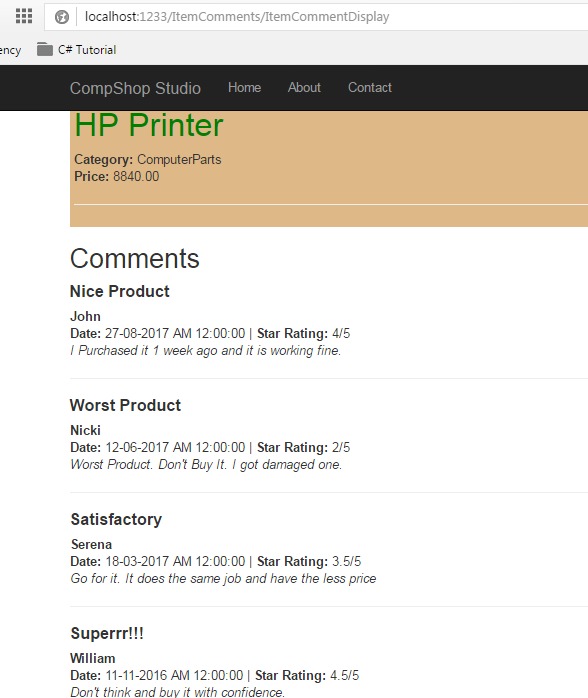
TempData
TempData is also used for transferring Models/Controllers data to views but it provides more functionality than ViewBag and ViewData. TempData doesn’t become null when redirection occurs and it is accessible in two continuous requests. However, TempData is mostly used for displaying Error Messages and Validation Messages. You must check for null and typecast complex data type in order to avoid errors.
ItemCommentsController.cs
and add the following highlighted action method.public ActionResult ItemCommentDisplay() { TempData["Item"] = GetItemDetails(); TempData["Comments"] = GetCommentList(); return View(); }
ItemCommentsController.cs
and change the ItemCommentDisplay()
action method.@using CompShop.Models @{ ItemModel Item = TempData["Item"] as ItemModel; List<BuyersCommentsModel> Comments = TempData["Comments"] as List<BuyersCommentsModel>; } <div> <h4><b>Pass Data using ViewBag</b></h4> <hr /> <dl class="dl-horizontal"> </dl> </div> <p> <!-- ITEM DETAILS --> <div style="background-color:burlywood; padding:5px;"> @if (Item != null) { <h1 style="color:green">@Item.Name</h1> <b>Category:</b> @Item.Category<br /> <b>Price:</b> @Item.Price } else { <h3 style="color:red">Sorry! Item Not Found</h3> } <hr /> </div> <!-- LOADING COMMENTS --> <h2>Comments</h2> @if (Comments != null) { foreach (var comments in Comments) { <h4><b>@comments.CommentsTitle</b></h4> <b>@comments.BuyersName</b><br /> <b>Date:</b> @comments.Date <span>|</span> <b>Star Rating: </b>@comments.StarRating<span>/5</span> <br /> <i>@comments.Comments</i> <hr /> } } else { <h3 style="color:red">No Comments Found!</h3> } </p>
Output
http://localhost:1233/ItemComments/ItemCommentDisplay
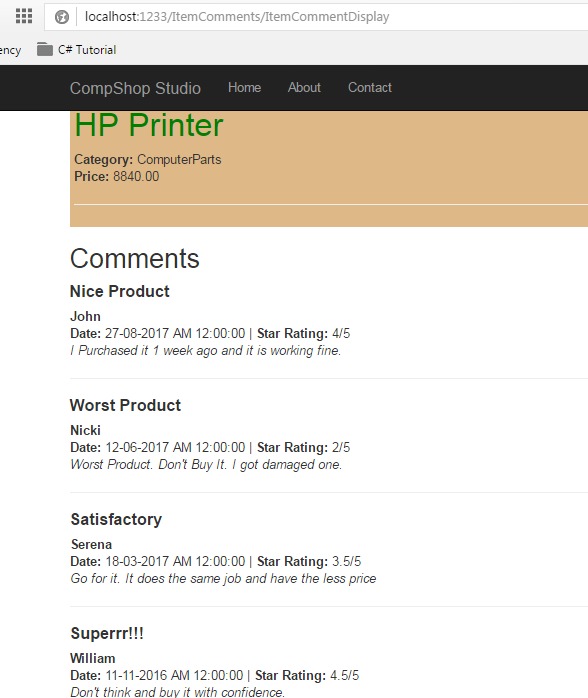
Summary
In this chapter, you learned how to pass multiple models data to view using ViewBag, ViewData and TempData. However, there are also various ways to achieve this goal and in the next chapter, you will learn How to use Session to Pass Model to Views.