1. What is
Html.RadioButton
and Html.RadioButtonFor
Methods?2. What is the difference between Html.RadioButton and Html.RadioButtonFor method?
3. How to grouping radio button in mvc?
4. Html.RadioButton Example
5. Html.RadioButtonFor Example
6. How to Bind Html.RadioButtonFor with a Model
7. How to send RadioButton Value to Controller
8. Html Attributes of Html.RadioButton
Radio Button provides list of options and lets you choose only one option in a panel. Radio Button is used widely in a form and carry true for selected items and false for non-selected items. Html.Helper class provides 2 extension methods to work with Radio Button; Html.RadioButton
and Html.RadioButtonFor
method.
@Html.RadioButton
Html.RadioButton
is loosely typed method which is not restricted to a model property. You must pass a string with the same name as a model property in order to avoid the runtime error.
RadioButton(string name, object value, bool isChecked, object htmlAttributes)
String
name : It is a group name. Object
value: This value will be posted to server if radio button is selected.Bool
isChecked: Set default radio button selectionObject
HtmlAttributes: Set additional properties to a radio button.Example:
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public string TeaType { get; set; } public string HotelType { get; set; } } }
Index.cshtml
@model HtmlHelperDemo.Models.UserModel @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Select Tea Type: </b><br /> <span>Tea: </span> @Html.RadioButton("TeaType","Tea",false) <span> | Coffee: </span> @Html.RadioButton("TeaType", "Coffee",true) <span> | BlackTea: </span> @Html.RadioButton("TeaType", "BlackTea",false) <span> | GreenTea: </span> @Html.RadioButton("TeaType", "GreenTea", false) <br /> <hr /> <b>Select Hotel: </b><br /> <span>Hotel Grand Plaza: </span> @Html.RadioButton("HotelType","HotelGrandPlaza", false) <span> | Hotel Lake View: </span> @Html.RadioButton("HotelType", "HotelLakeView", false) <span> | Hotel River Side: </span> @Html.RadioButton("HotelType", "HotelRiverSide", true) <span> | Hotel Mountain View: </span> @Html.RadioButton("HotelType", "MountainView", false) <br /> <input type="submit" value="submit" /> } <h4>You Selected</h4> <b>Tea Type: </b>@ViewBag.TeaType<br /> <b>Hotel Type: </b>@ViewBag.HotelType
HomeController.cs
[HttpPost] public ActionResult Index(UserModel u) { ViewBag.TeaType = u.TeaType.ToString(); ViewBag.HotelType = u.HotelType.ToString(); return View(); }
Html Output:
<input id="TeaType" name="TeaType" type="radio" value="Tea" />
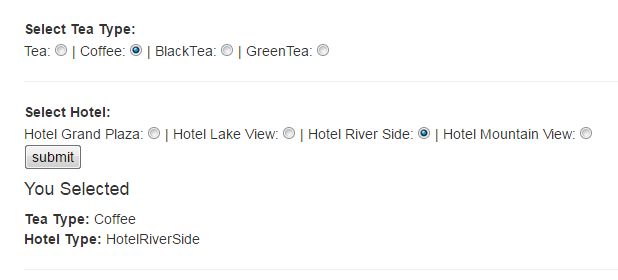
@Html.RadioButtonFor
Html.RadioButtonFor
method is strongly typed method which is restricted to a model property. It checks for all the errors at compile time and saves your application from getting any runtime error.
Html.RadioButtonFor(model => property, object value, object htmlAttributes)
Model
=> property: it refers to a model property.Object
value: This value will be posted to server if radio button is selected.Object
HtmlAttributes: Set additional properties to a radio button.UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public string TeaType { get; set; } public string HotelType { get; set; } } }
Index.cshtml
@model HtmlHelperDemo.Models.UserModel @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Select Tea Type: </b><br /> <span>Tea: </span> @Html.RadioButtonFor(m => m.TeaType, "Tea") <span> | Coffee: </span> @Html.RadioButtonFor(m => m.TeaType, "Coffee") <span> | BlackTea: </span> @Html.RadioButtonFor(m => m.TeaType, "BlackTea") <span> | GreenTea: </span> @Html.RadioButtonFor(m => m.TeaType, "GreenTea") <br /> <hr /> <b>Select Hotel: </b><br /> <span>Hotel Grand Plaza: </span> @Html.RadioButtonFor(m => m.HotelType, "HotelGrandPlaza") <span> | Hotel Lake View: </span> @Html.RadioButtonFor(m => m.HotelType, "HotelLakeView") <span> | Hotel River Side: </span> @Html.RadioButtonFor(m => m.HotelType, "HotelRiverSide") <span> | Hotel Mountain View: </span> @Html.RadioButtonFor(m => m.HotelType, "MountainView") <br /> <input type="submit" value="submit" /> } <h4>You Selected</h4> <b>Tea Type: </b>@ViewBag.TeaType<br /> <b>Hotel Type: </b>@ViewBag.HotelType
HomeController.cs
[HttpPost] public ActionResult Index(UserModel u) { ViewBag.TeaType = u.TeaType.ToString(); ViewBag.HotelType = u.HotelType.ToString(); return View(); }
Html Output
<input id="TeaType" name="TeaType" type="radio" value="Tea" />
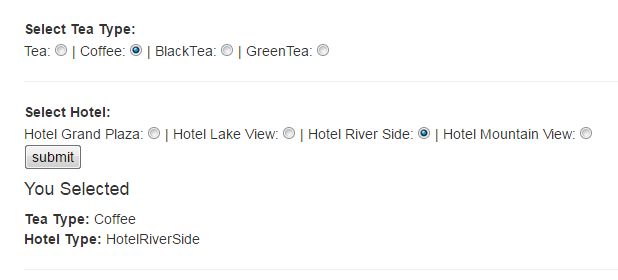
Html Attributes
Html Attributes set Html Properties for input control like width, color, value, css class etc. Attributes provides additional information about elements and always comes with name="value" pair.
<b>Tea: </b> @Html.RadioButtonFor(m => m.TeaType, "Tea", new { @value = "Tea", @class = "red", //@disabled="true", @checked = "true", @title = "Select, If you want tea.", @tabindex = "0", //@accesskey="z", @align = "left", @autofocus = "true", @style = "background-color:yellow; font-weight:bold", @draggable = "true", //@hidden="true", //@maxlength="12", })
Html Output
<b>Tea: </b><input align="left" autofocus="true" checked="true" class="red" draggable="true" id="TeaType" name="TeaType" style="background-color:yellow; font-weight:bold" tabindex="0" title="Select, If you want tea." type="radio" value="Tea" />
Difference Between Html.RadioButton
and Html.RadioButtonFor
method.
Html.RadioButton() | Html.RadioButtonFor() |
---|---|
It is loosely typed. It may be or not bounded with Model Properties. | It is strongly typed. Means, It will be always bounded with a model properties. |
It requires property name as string. | It requires property name as lambda expression. |
It doesn’t give you compile time error if you have passed incorrect string as parameter that does not belong to model properties. | It checks controls at compile time and if any error found it raises error. |
It throws run time error. Run time error gives bad impression to user and if the project is worthy, you may lose your client simply because of one error. | It throws compile time error which can be corrected before launching the project. It enhances user experience without throwing error. |
Summary
In this chapter, you learned Html.RadioButton()
and Html.RadioButtonFor
Helper Extension method in ASP.NET MVC 5 with complete programming example. I kept this chapter simple, short and easy; so hopefully, you will not get any problem in understanding this chapter. In the next chapter, you will learn about Html.DropDownList() extension method.