1. What are Session, Dynamic and Tuples in ASP.NET MVC?
2. Session Examples in MVC
3. Dynamic Examples in MVC
4. Tuples Examples in MVC
In the previous chapter, you learned how to use ViewBag, ViewData, and TempData to pass information from models/controllers to View Page. In this chapter, you are going to learn Session, Dynamic and Tuples with programming example.
Programming Examples of Session, Tuples, and Dynamic (ExpandoObject)
Before Starting this section, you must create following model classes and controllers. If you have already created it in the previous chapter then skip creating these models and controllers.
Using System; namespace CompShop.Models { public class ItemModel { public int ID { get; set; } public string Name { get; set; } public string Category { get; set; } public decimal Price { get; set; } } }
BuyersCommentsModel – It retrieves Comments
using System; namespace CompShop.Models { public class BuyersCommentsModel { public int BuyersID { get; set; } public string BuyersName { get; set; } public string StarRating { get; set; } public string CommentsTitle { get; set; } public string Comments { get; set; } public DateTime Date { get; set; } } }
ItemCommentsController – It will retrieve Items and Comments from models. I have added some items and comments in controller class in order to reduce complexity. Using the same method you can display database value to view pages.
using System; using System.Collections.Generic; using System.Web.Mvc; using CompShop.Models; namespace CompShop.Controllers { public class ItemCommentsController : Controller { // GET: ItemComments public ActionResult Index() { return View(); } public ItemModel GetItemDetails() { ItemModel iModel = new ItemModel() { ID = 1, Name = "HP Printer", Category = "ComputerParts", Price = 8840 }; return iModel; } public List<BuyersCommentsModel> GetCommentList() { List<BuyersCommentsModel> CommentsList = new List<BuyersCommentsModel>(); CommentsList.Add(new BuyersCommentsModel() { BuyersID = 1, BuyersName = "John", StarRating = "4", CommentsTitle = "Nice Product", Comments = "I Purchased it 1 week ago and it is working fine.", Date = Convert.ToDateTime("27 August 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=2, BuyersName="Nicki", StarRating="2", CommentsTitle="Worst Product", Comments="Worst Product. Don't Buy It. I got damaged one.", Date=Convert.ToDateTime("12 June 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=3, BuyersName="Serena", StarRating="3.5", CommentsTitle="Satisfactory", Comments="Go for it. It does the same job and have the less price", Date=Convert.ToDateTime("18 March 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=4, BuyersName="William", StarRating="4.5", CommentsTitle="Superrr!!!", Comments="Don't think and buy it with confidence.", Date=Convert.ToDateTime("11 November 16") }); return CommentsList; } public ActionResult ItemCommentDisplay() { } } }
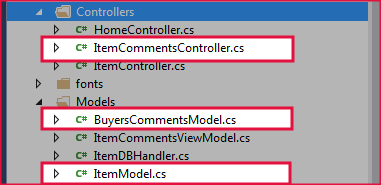
What is Session in ASP.NET MVC with Example
ASP.NET Session is also used for passing data between models/controllers to the view page but its value doesn’t become null after passing information until you manually set it to null or session times expires. By default, in ASP.NET MVC, session time is 20 minutes and you can increase or decrease time upon your needs. A session is mostly used in login page where a user is considered as validated until the session expires or the user clicks on log out button.
ItemCommentsController.cs
and add the following highlighted action method.public ActionResult ItemCommentDisplay() { Session["Item"] = GetItemDetails(); Session["Comments"] = GetCommentList(); return View(); }
ItemCommentDisplay()
Action Method and create a View.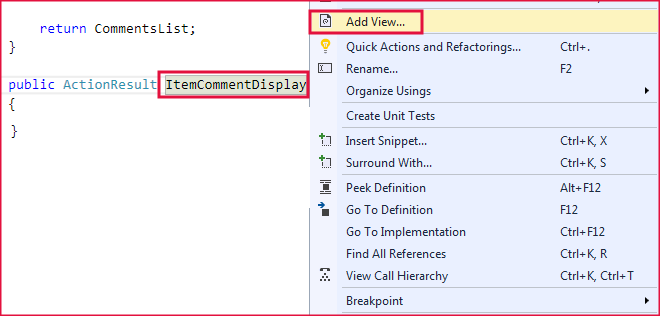
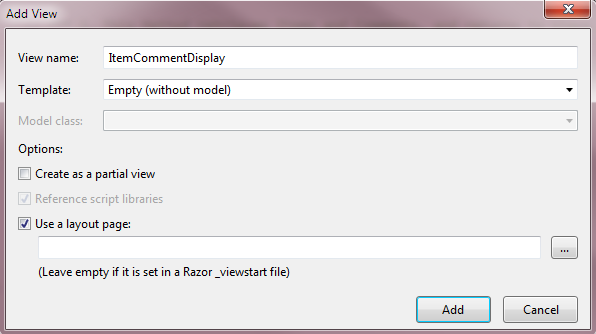
ItemCommentDisplay.cshtml
View Page.@using CompShop.Models @{ ItemModel Item = Session["Item"] as ItemModel; List<BuyersCommentsModel> Comments = Session["Comments"] as List<BuyersCommentsModel>; } <!-- ITEM DETAILS --> <div style="background-color:burlywood; padding:5px;"> @if (Item != null) { <h1 style="color:green">@Item.Name</h1> <b>Category:</b> @Item.Category<br /> <b>Price:</b> @Item.Price } else { <h3 style="color:red">Sorry! Item Not Found</h3> } <hr /> </div> <!-- LOADING COMMENTS --> <h2>Comments</h2> @if (Comments != null) { foreach (var comments in Comments) { <h4><b>@comments.CommentsTitle</b></h4> <b>@comments.BuyersName</b><br /> <b>Date:</b> @comments.Date <span>|</span> <b>Star Rating: </b>@comments.StarRating<span>/5</span> <br /> <i>@comments.Comments</i> <hr /> } } else { <h3 style="color:red">No Comments Found!</h3> }
Output
http://localhost:1233/ItemComments/ItemCommentDisplay
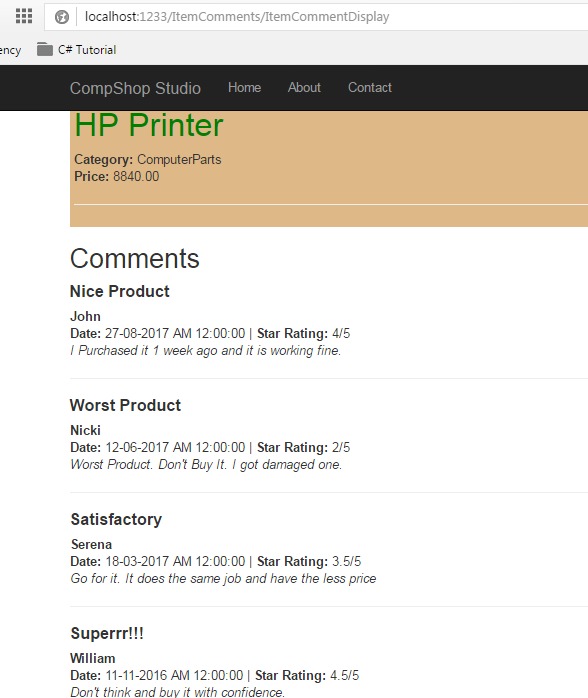
Tuples - What are Tuples in ASP.NET MVC with Example
Tuples are first introduced in .NET Framework 4.0. It is immutable, fixed-size and ordered sequence object. It is a data structure that has a specific number and sequence of elements and it supports up to 7 to 8 elements. You can use Tuples for passing multiple models in a single view page.
ItemCommentsController.cs
and change the ItemCommentDisplay()
action method.public ActionResult ItemCommentDisplay() { var commentdisplay = new Tuple<ItemModel, List<BuyersCommentsModel>>(GetItemDetails(), GetCommentList()); return View(commentdisplay); }
Let’s understand this code
As we discussed earlier that tuples supports maximum of 7 to 8 items; so pass the models in Tuple<model1, model2, model3,….model7>
brackets and then pass the respective action method in sequence that is mapped with model, like (function1, function2, function3,….function7)
. Here, I did the same thing. Just look this code below. Here I have passed my models and their respective action method in sequence.
var commentdisplay = new Tuple<ItemModel, List<BuyersCommentsModel>>(GetItemDetails(), GetCommentList());
ItemCommentDisplay.cshtml
View Page.@using CompShop.Models @model Tuple<ItemModel, List<BuyersCommentsModel>> <div> <h4><b>Pass Data using Tuples</b></h4> <hr /> <dl class="dl-horizontal"> </dl> </div> <p> <!-- ITEM DETAILS --> <div style="background-color:burlywood; padding:5px;"> <h1 style="color:green">@Model.Item1.Name</h1> <b>Category:</b> @Model.Item1.Category<br /> <b>Price:</b> @Model.Item1.Price <hr /> </div> <!-- LOADING COMMENTS --> <h2>Comments</h2> @foreach (var comments in Model.Item2) { <h4><b>@comments.CommentsTitle</b></h4> <b>@comments.BuyersName</b><br /> <b>Date:</b> @comments.Date <span>|</span> <b>Star Rating: </b>@comments.StarRating<span>/5</span> <br /> <i>@comments.Comments</i> <hr /> } </p>
Let me highlight some point of this code.
1. Add tuples in the beginning of the view page and pass models name in parameter.
@model Tuple<ItemModel, List<BuyersCommentsModel>>
2. You don’t need to remember the models name when accessing its member. You need to use
@Model.Item1, @Model.Item2, @Model.Item3…. @Model.Item7
for accessing the model. Here, @Model.Item1
represents ItemModel
, and @Model.Item2
represents List<BuyersCommentsModel>
http://localhost:1233/ItemComments/ItemCommentDisplay
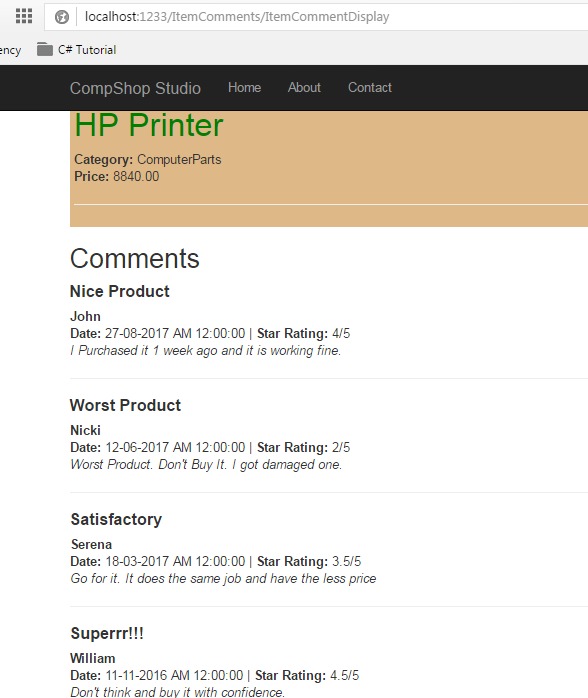
Dynamic (ExpandoObject) - Example
ExpandoObject
can also be used for passing data among Models, Controllers and Views. The member of ExpandoObject can be added or removed at runtime. It is very flexible and dynamic in nature and very useful when you want to display view page dynamically at runtime. We will discuss on Dynamic (ExpandoObject) in details later. Here, just see how to use Dynamic (ExpandoObject) for passing information in MVC.
ItemCommentsController.cs
and change the ItemCommentDisplay()
action method.public ActionResult ItemCommentDisplay() { dynamic cmnt = new ExpandoObject(); cmnt.Item = GetItemDetails(); cmnt.Comments = GetCommentList(); return View(cmnt); }
ItemCommentDisplay.cshtml
View Page.@using CompShop.Models @model dynamic <div> <h4><b>Pass Data using Dynamic</b></h4> <hr /> <dl class="dl-horizontal"> </dl> </div> <p> <!-- ITEM DETAILS --> <div style="background-color:burlywood; padding:5px;"> <h1 style="color:green">@Model.Item.Name</h1> <b>Category:</b> @Model.Item.Category<br /> <b>Price:</b> @Model.Item.Price <hr /> </div> <!-- LOADING COMMENTS --> <h2>Comments</h2> @foreach (var comments in Model.Comments) { <h4><b>@comments.CommentsTitle</b></h4> <b>@comments.BuyersName</b><br /> <b>Date:</b> @comments.Date <span>|</span> <b>Star Rating: </b>@comments.StarRating<span>/5</span> <br /> <i>@comments.Comments</i> <hr /> } </p>
http://localhost:1233/ItemComments/ItemCommentDisplay
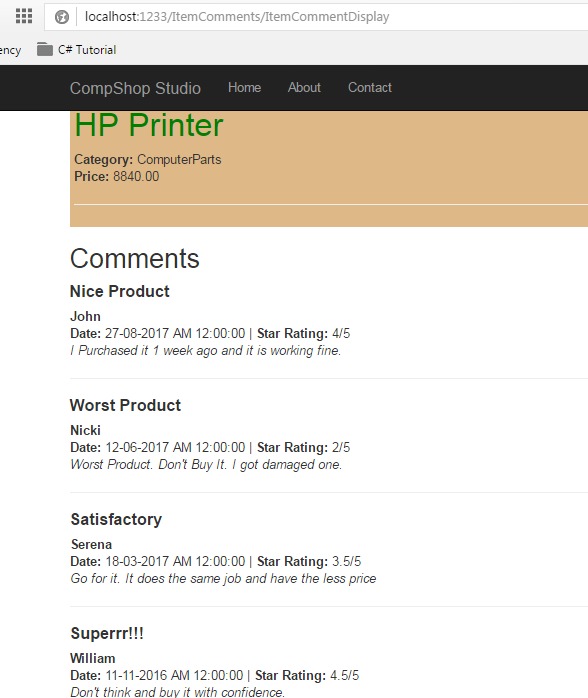
Summary
In this chapter, you learned how to pass multiple model data to views using Session, Tuples and Dynamic (ExpandoObject) in ASP.NET MVC. I tried to teach you these objects with the help of an easy and complete example. In the next chapter, you will learn how to pass multiple model data to a single view page using Render Action, JSON, and Navigation Property.