In this chapter, you will learn:
- What is
Html.ListBoxFor
Extension Methods? Html.ListBoxFor
Example- How Bind Html.ListBoxFor with a Model
- How to display List item in Html.ListBoxFor control
- How to send ListBoxFor Value to Controller
- Html Attributes of Html.ListBoxFor
What is Html.ListBoxFor Extension Method?
Html.ListBoxFor
Extension Method creates ListBox control and allows users to select multiple options in one go. It is very useful when you want to get multiple options for the users. The benefit of using ListBoxFor is, all the options visually appear and a user has the option to select one option as well as more than one options. Here, in this tutorial, I will explain, how to create ListBox in ASP.NET MVC using List Items and then post selected items to controllers.
How to Define Html.ListBoxFor input control?
You can define this control as follows:@Html.ListBoxFor(model => model.property, ItemList, object HtmlAttribute)
Model => model.property: It contains the ID of selected items
ItemList: It is a list of items
Object HtmlAttributes: It sets extra attributes to ListBox Control
Programming Example
Here, I am going to create a TeaList and later I will use this TeaList to show in ListBox and ask the user to select their choice.Model: UserModel.cs
using System.Collections.Generic; using System.Web.Mvc; namespace HtmlHelperDemo.Models { public class UserModel { public int[] SelectedTeaIds { get; set; } public IEnumerable<SelectListItem> TeaList { get; set; } } }
Controller: HomeController.cs
using System.Web.Mvc; using HtmlHelperDemo.Models; using System.Collections.Generic; using System.Linq; namespace HtmlHelperDemo.Controllers { public class HomeController : Controller { public ActionResult Index() { var model = new UserModel { SelectedTeaIds = new[] { 2 }, TeaList = GetAllTeaTypes() }; return View(model); } [HttpPost] public ActionResult Index(UserModel model) { model.TeaList = GetAllTeaTypes(); if(model.SelectedTeaIds!=null) { List<SelectListItem> selectedItems = model.TeaList.Where(p => model.SelectedTeaIds.Contains(int.Parse(p.Value))).ToList(); foreach (var Tea in selectedItems) { Tea.Selected = true; ViewBag.Message += Tea.Text + " | "; } } return View(model); } public List<SelectListItem> GetAllTeaTypes() { List<SelectListItem> items = new List<SelectListItem>(); items.Add(new SelectListItem { Text = "General Tea", Value = "1" }); items.Add(new SelectListItem { Text = "Coffee", Value = "2" }); items.Add(new SelectListItem { Text = "Green Tea", Value = "3" }); items.Add(new SelectListItem { Text = "Black Tea", Value = "4" }); return items; } } }
View: Index.cshtml
@using HtmlHelperDemo.Models @model UserModel <br /><br /> @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Select Tea Type: </b><br /> @Html.ListBoxFor(x => x.SelectedTeaIds, Model.TeaList, new { style= "width:200px"}) <br /> <input type="submit" value="submit" /> } <h4>You Selected</h4> <b style="color:red">Tea Type: @ViewBag.Message</b>Output:
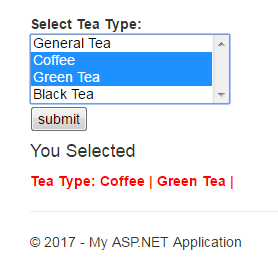
Html Attributes of Html.ListBoxFor
Html Attributes set Html Properties for input control like width, color, value, CSS class etc. Attributes provide additional information about elements and always comes with name="value" pair.@Html.ListBoxFor(x => x.SelectedTeaIds, Model.TeaList, new { @style = "width:200px; background-color:yellow; padding:15px;", @title = "Select Multiple Options", @align = "left", })Html Output