1. What are HTTPGET and HTTPPOST Method in MVC?
2. What are the differences between HttpGet and HttpPost Method?
3. Learn HttpGet with Programming Example
4. Learn HttpPost with Programming Example
HttpGet and HttpPost, both are the method of posting client data or form data to the server. HTTP is a HyperText Transfer Protocol that is designed to send and receive data between client and server using web pages. HTTP has two methods that are used for posting data from web pages to the server. These two methods are HttpGet and HttpPost.
HttpGet
HttpGet method sends data using a query string. The data is attached to URL and it is visible to all the users. However, it is not secure but it is fast and quick. It is mostly used when you are not posting any sensitive data to the server like username, password, credit card info etc.
- It is fast and quick but not secure.
- It is default method.
- Because it attached form data in query string, so the data is visible to other users.
- It uses stack method for passing form variable.
- Data is limited to max length of query string.
- It is very useful when data is not sensitive.
- It creates URL that is easily readable.
- It can carry only text data.
StudentModel.cs
namespace MvcForms.Models { public class StudentModel { public int Id { get; set; } public string Name { get; set; } } }
Index.cshtml
Form<h3><b>Forms - HTTPGET Method</b></h3> @using (Html.BeginForm("Submit", "Home", FormMethod.Get)) { <table> <tr> <td>Enter ID: </td> <td>@Html.TextBox("Id")</td> </tr> <tr> <td>Enter Name: </td> <td>@Html.TextBox("Name")</td> </tr> <tr> <td colspan="2"><input type="submit" value="Submit"></td> </tr> </table> } <h4 style="color:purple"> ID: @ViewBag.Id<br /> Name: @ViewBag.Name<br /> </h4>
HomeController
[HttpGet] public ActionResult Submit(int id, string name) { ViewBag.Id = id; ViewBag.Name = name; return View("Index"); }
When you will run the application and pass value using HttpGet method, you will see that data is attached to URL using Query String.
http://localhost:2709/Home/Submit?Id=1&Name=Diablo
In this previous link, the data is passed using query string because submit button used HttpGet Method to post data. You can see that Id=1&Name=Diablo in URL.
Output Image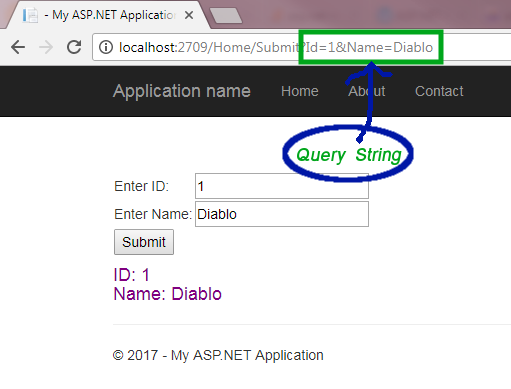
HttpPost
HTTPPost method hides information from URL and does not bind data to URL. It is more secure than HttpGet method but it is slower than HttpGet. It is only useful when you are passing sensitive information to the server.
- Data is sent via HttpPost method, is not visible to user.
- It is more secured but slower than HttpGet.
- It uses Heap method for passing form variable.
- It has no restriction of passing data and can post unlimited form variables.
- It is used when sending critical data.
- It can carry both text and binary data.
StudentModel.cs
namespace MvcForms.Models { public class StudentModel { public int Id { get; set; } public string Name { get; set; } } }
Index.cshtml
Form<h3><b>HTTPPost Method</b></h3> @using (Html.BeginForm("Submit", "Home", FormMethod.Post)) { <table> <tr> <td>Enter ID: </td> <td>@Html.TextBox("Id")</td> </tr> <tr> <td>Enter Name: </td> <td>@Html.TextBox("Name")</td> </tr> <tr> <td colspan="2"><input type="submit" value="Submit"></td> </tr> </table> } <h4 style="color:purple"> ID: @ViewBag.Id<br /> Name: @ViewBag.Name<br /> </h4>
HomeController.cs
[HttpPost] public ActionResult Submit(FormCollection fc) { ViewBag.Id = fc["Id"]; ViewBag.Name = fc["Name"]; return View("Index"); }
Look at this URL structure. You will notice that there is no data attached to the URL.
http://localhost:2709/Home/SubmitOutput
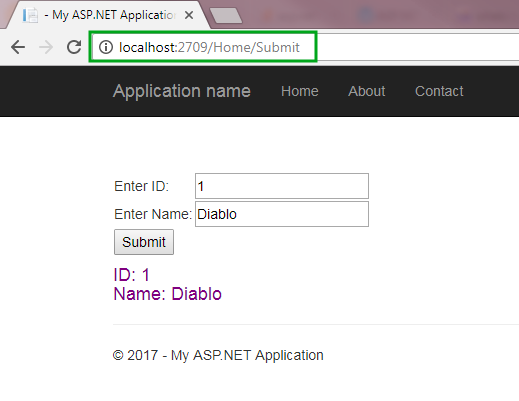
HTTPGet
method is default whereas you need to specify HTTPPost
attribute if you are posting data using HTTPPost
method.2.
HTTPGet
method creates a query string of the name-value pair whereas HTTPPost
method passes the name and value pairs in the body of the HTTP request.3.
HTTPGet
request has limited length and mostly it is limited to 255 characters long whereas HTTPPost
request has no maximum limit.4.
HTTPGet
is comparatively faster than HTTPPost
. HTTPPost
takes extra time in encapsulating the data.5.
HTTPGet
can carry only string data whereas HTTPPost
can carry both string and binary data.6.
HTTPGet
method creates readable url so it can be cached and bookmarked whereas such facility is not available in HTTPPost
method.Summary
In this chapter, I tried to explain HTTPGET and HTTPPOST method with an example. I have also explained the fact and differences between these two methods. Hope, now you are able to understand where to use HttpGet and HttpPost method. In the next chapter, you will learn Model Binding in ASP.NET MVC 5.