1. What is Render Action and Navigation Property in MVC
2. Learn Render Action by Example
3. Learn Navigation Property by Example
In the previous chapter, you learned how to pass data from models to views using Session, Tuples and Dynamic (ExpandoObject). In this chapter, you will learn to pass data among models, controllers, and views using Render Action and Navigation Property in ASP.NET MVC.
Before Starting this section, you must create following model classes and controllers. If you have already created it in the previous chapter then skip creating these models and controllers.
ItemModels – It retrieves Item Details
Using System; namespace CompShop.Models { public class ItemModel { public int ID { get; set; } public string Name { get; set; } public string Category { get; set; } public decimal Price { get; set; } } }
BuyersCommentsModel – It retrieves Comments
using System; namespace CompShop.Models { public class BuyersCommentsModel { public int BuyersID { get; set; } public string BuyersName { get; set; } public string StarRating { get; set; } public string CommentsTitle { get; set; } public string Comments { get; set; } public DateTime Date { get; set; } } }
ItemCommentsController – It will retrieve Items and Comments from models. I have added some items and comments in controller class in order to reduce complexity. Using the same method you can display database value to view pages.
using System; using System.Collections.Generic; using System.Web.Mvc; using CompShop.Models; namespace CompShop.Controllers { public class ItemCommentsController : Controller { // GET: ItemComments public ActionResult Index() { return View(); } public ItemModel GetItemDetails() { ItemModel iModel = new ItemModel() { ID = 1, Name = "HP Printer", Category = "ComputerParts", Price = 8840 }; return iModel; } public List<BuyersCommentsModel> GetCommentList() { List<BuyersCommentsModel> CommentsList = new List<BuyersCommentsModel>(); CommentsList.Add(new BuyersCommentsModel() { BuyersID = 1, BuyersName = "John", StarRating = "4", CommentsTitle = "Nice Product", Comments = "I Purchased it 1 week ago and it is working fine.", Date = Convert.ToDateTime("27 August 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=2, BuyersName="Nicki", StarRating="2", CommentsTitle="Worst Product", Comments="Worst Product. Don't Buy It. I got damaged one.", Date=Convert.ToDateTime("12 June 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=3, BuyersName="Serena", StarRating="3.5", CommentsTitle="Satisfactory", Comments="Go for it. It does the same job and have the less price", Date=Convert.ToDateTime("18 March 17") }); CommentsList.Add(new BuyersCommentsModel() {BuyersID=4, BuyersName="William", StarRating="4.5", CommentsTitle="Superrr!!!", Comments="Don't think and buy it with confidence.", Date=Convert.ToDateTime("11 November 16") }); return CommentsList; } public ActionResult ItemCommentDisplay() { } } }
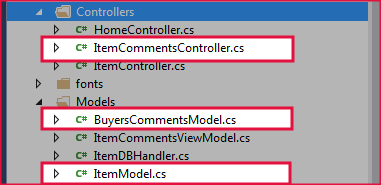
Render Action
What is Render ActionRender Action is a special controller method that includes partial view into main view page. If you want to display multiple models into a single view page, you can use Render Action Methods to do it.
Step 1: Create partial page for each models and display models information on that partial page.
Step 2: Now call all those partial pages inside a main view page using Render Action Method.
Render Action Example
ItemCommentsController.cs
and add the following highlighted action method.public ActionResult ItemCommentDisplay() { return View(); } public PartialViewResult RenderItem() { return PartialView(GetItemDetails()); } public PartialViewResult RenderComment() { return PartialView(GetCommentList()); }
RenderItem()
and select Add View.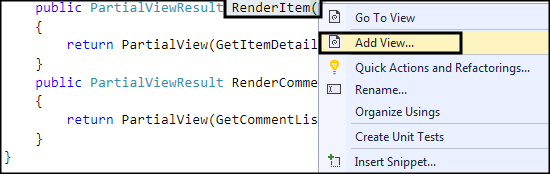
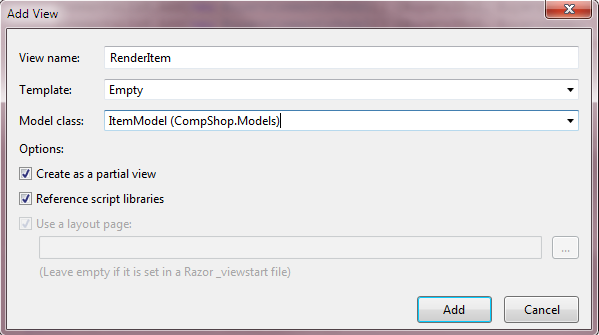
RenderItem.cshtml
partial view and Add the following code in it.@model CompShop.Models.ItemModel <div style="background-color:burlywood; padding:5px;"> <h1 style="color:green">@Model.Name</h1> <b>Category:</b> @Model.Category<br /> <b>Price:</b> @Model.Price <hr /> </div>
RenderComment()
Action method.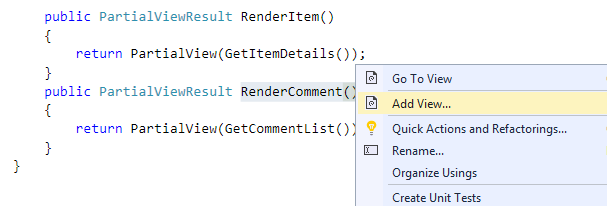
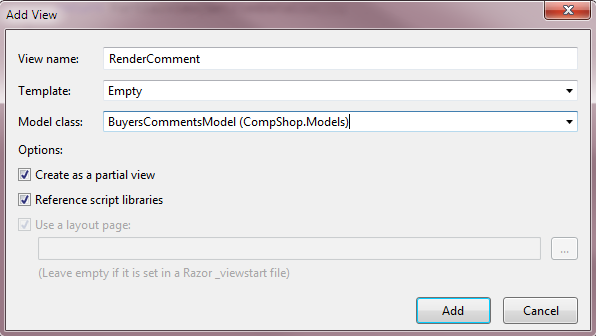
ItemCommentDisplay()
Action Method. Right click on ItemCommentDisplay()
Action method and click Add View.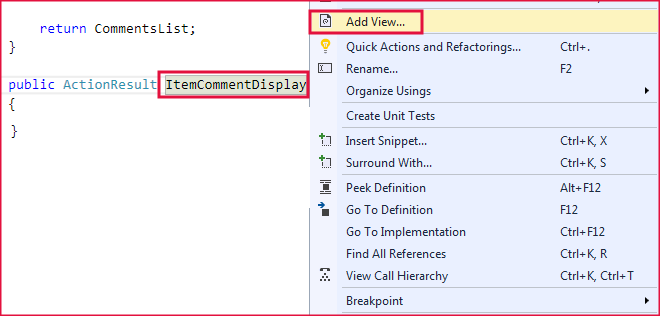
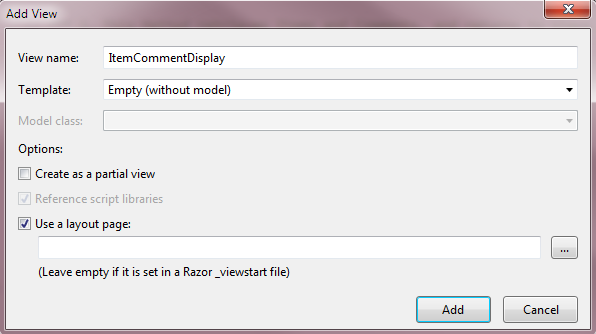
ItemCommentDisplay.cshtml
page.@{ Html.RenderAction("RenderItem"); Html.RenderAction("RenderComment"); }
http://localhost:1233/ItemComments/ItemCommentDisplay
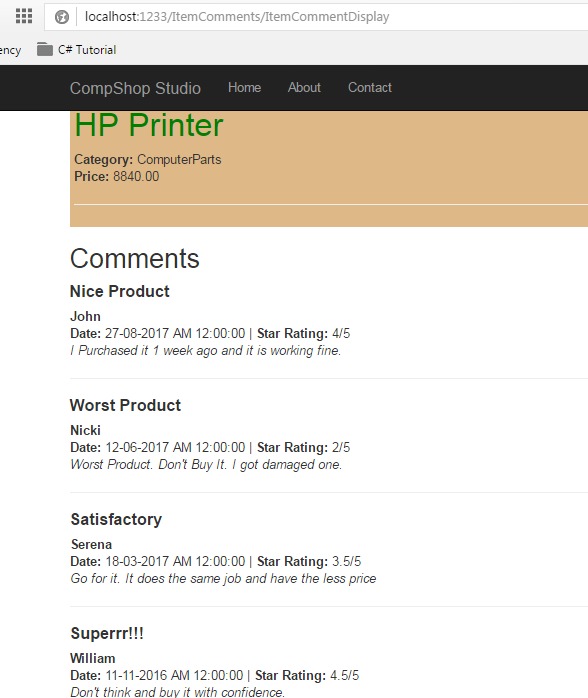
Navigation Property
Navigation Property binds two or more related models. If two models are related then you can bind one model into another model as property. By binding models as property, you can access both models member by calling only parent model.
Here, I have two models:1. ItemModel
2. BuyersCommentsModel
I will bind
BuyersCommentsModel
as property inside ItemModel
.
ItemModel
and insert the following line of code:using System; using System.Collections.Generic; namespace CompShop.Models { public class ItemModel { public int ID { get; set; } public string Name { get; set; } public string Category { get; set; } public decimal Price { get; set; } //Adding Navigation Property public List<BuyersCommentsModel> Comments { get; set; } } }
ItemCommentController.cs
and update highlighted line in GetItemDetails()
and ItemCommentDisplay()
Action Method.GetItemDetails
public ItemModel GetItemDetails()
{
ItemModel iModel = new ItemModel()
{
ID = 1,
Name = "HP Printer",
Category = "ComputerParts",
Price = 8840,
Comments = GetCommentList()
};
return iModel;
}
ItemCommentDisplay()
public ActionResult ItemCommentDisplay() { ItemModel Item = GetItemDetails(); return View(Item); }
ItemCommentDisplay.cshtml
page and update it with the following code.@model CompShop.Models.ItemModel <p> <!-- ITEM DETAILS --> <div style="background-color:burlywood; padding:5px;"> <h1 style="color:green">@Html.DisplayFor(i => i.Name)</h1> <b>Category:</b> @Html.DisplayFor(i => i.Category)<br /> <b>Price:</b> @Html.DisplayFor(i => i.Price) <hr /> </div> <!-- LOADING COMMENTS --> <h2>Comments</h2> @foreach (var comments in Model.Comments) { <h4><b>@comments.CommentsTitle</b></h4> <b>@comments.BuyersName</b><br /> <b>Date:</b> @comments.Date <span>|</span> <b>Star Rating: </b>@comments.StarRating<span>/5</span> <br /> <i>@comments.Comments</i> <hr /> } </p>
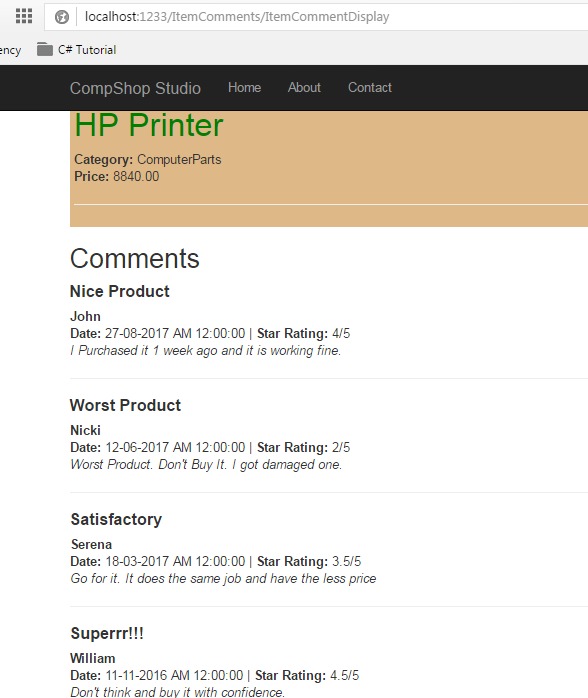
Summary
In this chapter, you learned how to pass multiple model data into a single view page using Render Action and Navigation Property. In this complete section, I taught you various ways to pass multiple model data into a single view page. I Hope that you will not face any problem in future when it comes to passing data between models and views. In the next chapter, I have added the very important topics of ASP.NET MVC and that is Working with Forms in ASP.NET MVC. If you cover the next chapter, you will be able to communicate with the database using forms in ASP.NET MVC.