1. What is
Html.CheckBox()
and Html.CheckBoxFor()
Methods?2. What is the difference between Html.CheckBox and Html.CheckBoxFor method?
3. Html.CheckBox Example
4. Html.CheckBoxFor Example
5. How to Bind Html.CheckBoxFor with a Model
6. How to send CheckBox Value to Controller?
7. Html Attributes of Html.CheckBox
A checkbox is a small box which lets you choose multiple options. It only carries true and false value for the checked and unchecked item. In ASP.NET MVC Helper class, you have given Html.CheckBox()
and Html.CheckBoxFor()
Extension method to work with. Here, in this article, we will learn both methods with complete programming example.
@Html.CheckBox: Example and Definition
Html.CheckBox()
is loosely typed method, which is not bounded by a model property. It doesn't check for error at compile time and if the problem arises, it raises errors at runtime.
CheckBox(string name, bool isChecked, object htmlAttributes)
String name is the name of the checkbox.
boolisChecked contains true or false value
HtmlAttributes allows you to add additional HTML properties
Example
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public bool Tea { get; set; } public bool Loundry { get; set; } public bool Breakfast { get; set; } } }
Index.cshtml
@using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Want Additional Benefit: </b><br /> <span>Tea: </span> @Html.CheckBox("Tea",true) <span> | Loundry: </span> @Html.CheckBox("Loundry", false) <span> | Breakfast: </span> @Html.CheckBox("Breakfast", false) }
Html Output
<span>Tea: </span> <input checked="checked" data-val="true" data-val-required="The Tea field is required." id="Tea" name="Tea" type="checkbox" value="true" /> <input name="Tea" type="hidden" value="false" /> <span> | Loundry: </span> <input data-val="true" data-val-required="The Loundry field is required." id="Loundry" name="Loundry" type="checkbox" value="true" /> <input name="Loundry" type="hidden" value="false" /> <span> | Breakfast: </span> <input data-val="true" data-val-required="The Breakfast field is required." id="Breakfast" name="Breakfast" type="checkbox" value="true" /> <input name="Breakfast" type="hidden" value="false" />
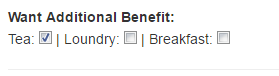
The Html.CheckBox extension method generates pure HTML checkbox with following values:
a. Id and name denote the Model Property name.
b. Type denotes that it is checkbox
c. Checked denotes whether a checkbox is selected or not d. Data-val ="true" and data-val-required="The Tea field is required." are coming because By default MVC adds [Required] attribute to non-nullable value types. To fix it, add the following line into
Application_Start
method in Global.asax file.
DataAnnotationsModelValidatorProvider.AddImplicitRequiredAttributeForValueTypes = false;
e.
<input name="Tea" type="hidden" value="false" />
You have noticed that @Html.CheckBox
is generating an additional hidden field with a false value. It is because, when you not select a checkbox, still form sends a false value to the server. It is the way of managing checkbox state in MVC.
@Html.CheckBoxFor: Example and Definition
Html.CheckBoxFor
is strongly bounded with model properties and checks for all errors at compile time. Before using this method, you must bind views with a model. Add a model name in the top of the view as follows:
@model HtmlHelperDemo.Models.UserModel
Define:
CheckBoxFor(model > Property, bool isChecked, object htmlAttributes)
Model > Property
is the model connection of this checkbox.isChecked
refers to the true/false valueHtmlAttributes
sets additional properties to the checkbox.Example
UserModel.cs
namespace HtmlHelperDemo.Models { public class UserModel { public bool Tea { get; set; } public bool Loundry { get; set; } public bool Breakfast { get; set; } } }
Index.cshtml
@model HtmlHelperDemo.Models.UserModel @using (Html.BeginForm("Index", "Home", FormMethod.Post)) { <b>Want Additional Benefit: </b><br /> <span>Tea: </span> @Html.CheckBoxFor(m => m.Tea, true) <span> | Loundry: </span> @Html.CheckBoxFor(m => m.Loundry, false) <span> | Breakfast: </span> @Html.CheckBoxFor(m => m.Breakfast, false) }
Html Output:
<span>Tea: </span> <input checked="checked" id="Tea" name="Tea" type="checkbox" value="true" /> <input name="Tea" type="hidden" value="false" /> <span> | Loundry: </span> <input checked="checked" id="Loundry" name="Loundry" type="checkbox" value="true" /> <input name="Loundry" type="hidden" value="false" /> <span> | Breakfast: </span> <input data-val="true" data-val-required="The Breakfast field is required." id="Breakfast" name="Breakfast" type="checkbox" value="true" /> <input name="Breakfast" type="hidden" value="false" />
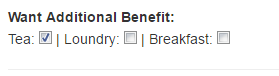
It generates the same HTML as @Html.CheckBox
generates.
How to send CheckBox Value to Controller
HomeController.cs
[HttpPost] public ActionResult Index(UserModel u) { ViewBag.Tea = u.Tea; ViewBag.Loundry = u.Loundry; ViewBag.Breakfast = u.Breakfast; return View(); }
Html Attributes
Html Attributes set Html Properties for input control like width, color, value, CSS class etc. Attributes provide additional information about elements and always comes with name="value" pair.
Example:@Html.CheckBoxFor(m => m.Tea, new { @value = "Tea", @class = "red", //@disabled="true", @checked = "true", @title = "Select, If you want tea.", @tabindex = "0", //@accesskey="z", @align = "left", @autofocus = "true", @style = "background-color:yellow; font-weight:bold", @draggable = "true", //@hidden="true", //@maxlength="12", })
Html Output:
<input align="left" autofocus="true" checked="true" class="red" draggable="true" id="Tea" name="Tea" style="background-color:yellow; font-weight:bold" tabindex="0" title="Select, If you want tea." type="checkbox" value="Tea" /><input name="Tea" type="hidden" value="false" />
Difference Between Html.CheckBox and Htm.CheckBoxFor method.
Html.CheckBox() | Html.CheckBoxFor() |
---|---|
It is loosely typed. It may be or not bounded with Model Properties. | It is strongly typed. Means, It will be always bounded with a model properties. |
It requires property name as string. | It requires property name as lambda expression. |
It doesn’t give you compile time error if you have passed incorrect string as parameter that does not belong to model properties. | It checks controls at compile time and if any error found it raises error. |
It throws run time error. Run time error gives bad impression to user and if the project is worthy, you may lose your client simply because of one error. | It throws compile time error which can be corrected before launching the project. It enhances user experience without throwing error. |
Summary
In this chapter, you learned Html.CheckBox()
and Html.CheckBoxFor()
Helper Extension method in ASP.NET MVC 5 with complete programming example. I kept this chapter simple, short and easy so hopefully you will not get any problem in understanding this chapter. In the next chapter, you will learn about Html.RadioButton() extension method.